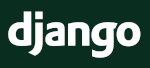
Web Server Gateway Interface (WSGI) is a specification that defines the communication between web servers and web applications in Python. Django, a high-level Python web framework, supports WSGI and provides tools to create robust web applications. This article provides an in-depth guide on writing a basic Django WSGI handler, explaining its components and their interactions.
Prerequisites
Before you begin, ensure you have the following:
- Python 3.6 or higher
- Django installed (version 3.0 or higher)
- Basic understanding of Django and WSGI concepts
Setting Up the Django Project
-
Install Django: If you haven't installed Django, use pip to install it.
pip install django
-
Create a Django Project:
django-admin startproject myproject cd myproject
-
Create a Django Application:
python manage.py startapp myapp
Understanding WSGI
WSGI is a Python standard described in PEP 3333. It serves as a bridge between web servers and Python web applications or frameworks. The core idea of WSGI is to separate web servers and web applications, allowing them to interact through a standard interface.
Basic WSGI Application
A minimal WSGI application is a callable object (like a function) that accepts
two arguments: environ
and start_response
.
environ
: A dictionary containing CGI-style environment variables.start_response
: A callback function to start the HTTP response.
Here’s a simple WSGI application example:
def simple_app(environ, start_response):
status = '200 OK'
headers = [('Content-Type', 'text/plain')]
start_response(status, headers)
return [b"Hello, World!"]
Django's WSGI Handler
Django includes a built-in WSGI handler that makes it easy to deploy Django
applications using any WSGI-compatible web server, such as Gunicorn or uWSGI. The
default WSGI handler is located in myproject/wsgi.py
.
wsgi.py
File
When you create a new Django project, the wsgi.py
file is generated
automatically. This file serves as the entry point for WSGI-compatible web
servers.
import os
from django.core.wsgi import get_wsgi_application
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'myproject.settings')
application = get_wsgi_application()
Breakdown of wsgi.py
-
Importing Necessary Modules:
import os from django.core.wsgi import get_wsgi_application
-
Setting the Default Django Settings Module:
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'myproject.settings')
This line sets the default settings module for the Django project. The settings module contains configuration for the Django project.
-
Creating the WSGI Application:
application = get_wsgi_application()
get_wsgi_application()
returns a WSGI callable that can be used by any WSGI server to forward requests to Django.
Customizing the WSGI Handler
Sometimes, you may need to customize the WSGI handler for additional functionality, such as middleware integration or request logging.
Adding Middleware
To add custom middleware, you can wrap the default Django WSGI application. Here’s an example of adding a simple middleware for logging requests:
-
Create a Middleware Module: Create a new file
myproject/middleware.py
and add the following code:class SimpleLoggingMiddleware: def __init__(self, app): self.app = app def __call__(self, environ, start_response): # Log request details print(f"Request: {environ['REQUEST_METHOD']} {environ['PATH_INFO']}") return self.app(environ, start_response)
-
Update
wsgi.py
:import os from django.core.wsgi import get_wsgi_application from .middleware import SimpleLoggingMiddleware os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'myproject.settings') application = get_wsgi_application() application = SimpleLoggingMiddleware(application)
Handling Custom Environments
You can also modify the WSGI handler to handle different environments, such as development, staging, or production. Here’s an example:
- Create Environment-Specific Settings:
- Create different settings files, e.g.,
settings_dev.py
,settings_prod.py
. - Modify
settings_dev.py
andsettings_prod.py
as needed. -
Update
wsgi.py
to Handle Environments:import os from django.core.wsgi import get_wsgi_application environment = os.environ.get('DJANGO_ENV', 'development') settings_module = f"myproject.settings_{environment}" os.environ.setdefault('DJANGO_SETTINGS_MODULE', settings_module) application = get_wsgi_application()
Deploying with a WSGI Server
To deploy the Django application, you can use any WSGI-compatible server. Here’s how to deploy with Gunicorn:
-
Install Gunicorn:
pip install gunicorn
-
Run the Application:
gunicorn myproject.wsgi:application
This command tells Gunicorn to serve the WSGI application defined in
myproject.wsgi
.
Conclusion
Creating a basic Django WSGI handler involves setting up a Django project, understanding the WSGI interface, and customizing the WSGI handler as needed. By following the steps outlined in this article, you can create and deploy a Django application using a WSGI-compatible server. This setup provides a flexible and scalable foundation for serving web applications in production environments.