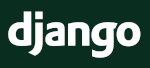
When developing web applications with Django, there are scenarios where you need to display data from multiple models within a single view. To achieve this, you combine the data from these models into one context variable. This article provides an in-depth guide on how to efficiently combine multiple models into one context variable and display them in your Django templates.
Prerequisites
Before we dive into the technical details, ensure you have the following:
- Python 3.6 or higher
- Django installed (version 3.0 or higher)
- Basic understanding of Django models, views, and templates
Understanding Context in Django
In Django, context is a dictionary that contains data passed from views to templates. This data can include variables, querysets, and more. The context dictionary is used to render dynamic content in templates.
Basic Context Example
Here’s a simple example of passing a context variable to a template:
# views.py
from django.shortcuts import render
def my_view(request):
context = {
'message': 'Hello, World!'
}
return render(request, 'my_template.html', context)
In the above example, the message
variable is passed to the my_template.html
template.
Combining Multiple Models
Scenario
Suppose we have two models, Author
and Book
, and we want to display a list of
authors and their books on the same page.
Models Definition
First, let's define our models in models.py
:
# models.py
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
def __str__(self):
return self.name
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
published_date = models.DateField()
def __str__(self):
return self.title
Creating the View
Next, we create a view that combines data from these two models into one context variable.
Approach 1: Using Separate QuerySets
One approach is to retrieve the data using separate querysets and combine them in the context.
# views.py
from django.shortcuts import render
from .models import Author, Book
def author_book_list(request):
authors = Author.objects.all()
books = Book.objects.all()
context = {
'authors': authors,
'books': books,
}
return render(request, 'author_book_list.html', context)
In this approach, we pass two querysets, authors
and books
, to the template.
Approach 2: Using a Single Context Variable
Alternatively, you can combine the data into a single context variable.
# views.py
from django.shortcuts import render
from .models import Author, Book
def author_book_list(request):
authors = Author.objects.all()
books = Book.objects.all()
author_books = []
for author in authors:
author_books.append({
'author': author,
'books': books.filter(author=author)
})
context = {
'author_books': author_books,
}
return render(request, 'author_book_list.html', context)
Using the Context in Templates
In the template, you can loop through the combined context variable to display the data.
<!-- author_book_list.html -->
<!DOCTYPE html>
<html>
<head>
<title>Authors and Books</title>
</head>
<body>
<h1>Authors and Books</h1>
{% for entry in author_books %}
<h2>{{ entry.author.name }}</h2>
<ul>
{% for book in entry.books %}
<li>{{ book.title }} ({{ book.published_date }})</li>
{% endfor %}
</ul>
{% endfor %}
</body>
</html>
In this template, we iterate over the author_books
context variable to display
each author and their respective books.
Advanced Techniques
Using Prefetch Related
For improved performance, especially when dealing with a large number of records,
you can use Django’s prefetch_related
to reduce the number of database queries.
# views.py
from django.shortcuts import render
from django.db.models import Prefetch
from .models import Author, Book
def author_book_list(request):
authors = Author.objects.prefetch_related(
Prefetch('book_set', queryset=Book.objects.all(), to_attr='books')
)
context = {
'authors': authors,
}
return render(request, 'author_book_list.html', context)
In this approach, the authors
queryset prefetches related books, reducing the
number of queries executed when accessing the books in the template.
Custom Template Tags
For more complex scenarios, creating custom template tags can be a powerful solution.
-
Create a Template Tag Module:
# templatetags/custom_tags.py from django import template from ..models import Book register = template.Library() @register.simple_tag def get_books_by_author(author): return Book.objects.filter(author=author)
-
Load and Use the Template Tag in Template:
<!-- author_book_list.html --> {% load custom_tags %} <!DOCTYPE html> <html> <head> <title>Authors and Books</title> </head> <body> <h1>Authors and Books</h1> {% for author in authors %} <h2>{{ author.name }}</h2> <ul> {% get_books_by_author author as books %} {% for book in books %} <li>{{ book.title }} ({{ book.published_date }})</li> {% endfor %} </ul> {% endfor %} </body> </html>
This approach keeps the view logic simple and delegates the data retrieval to the template.
Conclusion
Combining multiple models into one context variable in Django is a common
requirement when developing complex web applications. By understanding and
implementing the techniques discussed in this article, you can efficiently manage
and display data from multiple models in your Django views and templates. Whether
you use separate querysets, combine them into a single variable, leverage
prefetch_related
for performance, or create custom template tags, Django
provides the flexibility and tools to achieve your goals.
Further reading: