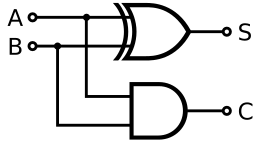
When you delve into the fascinating world of programming and software development, you may come across various terms and concepts that, at first, appear complex and mystifying. However, many of these seemingly intricate terms are often synonymous, and understanding their true meaning can simplify your journey as a coder. One such example is the relationship between "decision structures" and "selection structures."
Decision Structures and Selection Structures: Unraveling the Connection
At its core, both "decision structures" and "selection structures" share a common objective: to enable a program to make choices and take different actions based on certain conditions. These structures are the building blocks of creating responsive and dynamic software. The key distinction lies in the terminology, and it's essential to recognize that they essentially refer to the same concept.
Decision Structures
Decision structures are aptly named because they revolve around making decisions within your code. These decisions often hinge on the evaluation of a condition or a set of conditions. If the condition is met, the program executes one set of instructions; if not, it proceeds with an alternative set of instructions. This is the fundamental mechanism behind "if" statements in programming.
For instance, consider a simple decision structure in a Python program:
if user_age >= 18:
print("You are an adult.")
else:
print("You are not yet an adult.")
In this example, the decision structure evaluates whether the user's age is greater than or equal to 18, and it executes different print statements based on the outcome.
Selection Structures
On the other hand, selection structures are synonymous with decision structures. They are responsible for selecting one of multiple paths or courses of action based on the conditions provided. These structures include constructs like "if-else" statements, "switch-case" statements (in languages like C++ and Java), and other logical constructs used to select from among several options.
Here's a Java code snippet showcasing a "selection structure" using a "switch-case" statement:
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
// ... More cases ...
default:
dayName = "Unknown";
}
In this example, the selection structure, implemented with a "switch-case"
statement, selects the value of dayName
based on the value of the day
variable.
The Bottom Line
In the realm of programming, terminology can sometimes be perplexing, with different words used to describe similar concepts. Decision structures and selection structures exemplify this phenomenon. In essence, both terms refer to the process of making choices in your code based on conditions.
So, whether you're reading code, writing it, or discussing programming concepts with peers, remember that decision structures and selection structures are two sides of the same coin. They empower your code to be dynamic and responsive, enabling it to adapt to various scenarios, which is at the heart of effective software development.