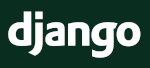
Combining Django's powerful back-end capabilities with jQueryUI's dynamic front-end features can create seamless and interactive web applications. One common requirement is to control which tab is open when a page loads, especially when you need to highlight a specific section based on user actions or URL parameters. This article provides an in-depth guide on how to achieve this using Django and jQueryUI Tabs.
Prerequisites
Before we start, ensure you have the following:
- Python 3.6 or higher
- Django installed (version 3.0 or higher)
- Basic understanding of Django views, templates, and static files
- Basic knowledge of HTML, CSS, and JavaScript
Setting Up Django
First, we need to set up a Django project and an application.
Step 1: Create a Django Project and Application
-
Install Django:
pip install django
-
Create a new Django project:
django-admin startproject myproject cd myproject
-
Create a new Django application:
python manage.py startapp myapp
-
Add the application to
INSTALLED_APPS
inmyproject/settings.py
:INSTALLED_APPS = [ ... 'myapp', ]
Step 2: Define Models (if needed)
For this tutorial, we won't need specific models, but in a real application, you might want to define models and use them in your views and templates.
Step 3: Create a View
Create a simple view in myapp/views.py
that renders a template with jQueryUI
Tabs.
# myapp/views.py
from django.shortcuts import render
def index(request):
return render(request, 'index.html')
Step 4: Set Up URLs
Update myproject/urls.py
to include the application’s URL.
# myproject/urls.py
from django.contrib import admin
from django.urls import path
from myapp import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index, name='index'),
]
Creating the Template
Step 5: Set Up the Template
Create an index.html
file in myapp/templates/
.
<!-- myapp/templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Django + jQueryUI Tabs</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script>
$(document).ready(function() {
var tabIndex = 0; // Default tab index
var urlParams = new URLSearchParams(window.location.search);
if (urlParams.has('tab')) {
tabIndex = parseInt(urlParams.get('tab'));
}
$("#tabs").tabs({
active: tabIndex
});
});
</script>
</head>
<body>
<div id="tabs">
<ul>
<li><a href="#tab-1">Tab 1</a></li>
<li><a href="#tab-2">Tab 2</a></li>
<li><a href="#tab-3">Tab 3</a></li>
</ul>
<div id="tab-1">
<p>Content for Tab 1.</p>
</div>
<div id="tab-2">
<p>Content for Tab 2.</p>
</div>
<div id="tab-3">
<p>Content for Tab 3.</p>
</div>
</div>
</body>
</html>
Explanation of the Template
- jQueryUI CSS and JS: We include the jQuery and jQueryUI libraries for tab functionality.
- JavaScript Logic:
- The script captures URL parameters to determine which tab should be active.
- The
tabs
method initializes the tabs and sets the active tab based on thetab
URL parameter.
Handling URL Parameters
Step 6: Open a Specific Tab Based on URL Parameter
When the page loads, the script checks for a tab
parameter in the URL. For
example, if the URL is http://localhost:8000/?tab=1
, the second tab (index 1)
will be open on page load.
Step 7: Generating URLs with Parameters in Django
To generate URLs with parameters dynamically in your Django views, you can use
the url
template tag or build URLs in your views.
Example with a Link in a Template
<a href="{% url 'index' %}?tab=1">Open Tab 2</a>
Example with URL Generation in a View
from django.shortcuts import redirect
from django.urls import reverse
def redirect_to_tab(request, tab_index):
url = f"{reverse('index')}?tab={tab_index}"
return redirect(url)
Conclusion
Integrating Django with jQueryUI Tabs to open a specific tab on page load involves a few key steps: setting up your Django project and application, creating a view and a template, and implementing JavaScript to handle URL parameters. This approach provides a flexible way to enhance user experience by dynamically controlling which tab is displayed based on user actions or specific conditions.
By following this guide, you can effectively combine the power of Django’s backend with jQueryUI’s frontend capabilities to create interactive and user-friendly web applications.
Further reading:
- Learn Python the Hard Way
- jQuery: Novice to Ninja (HIGHLY RECOMMENDED!)
- Django Documentation