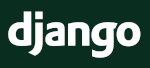
Sending emails is a crucial feature for web applications, whether it's for user registration, password recovery, or notifications. While developing a Django application, it's essential to configure and test email functionality in your development environment. This guide will walk you through setting up Django mailer in your local development setup.
Prerequisites
Ensure you have the following:
- Python 3.6 or higher
- Django installed (version 3.0 or higher)
Setting Up Email Backend
Step 1: Configure Email Settings
First, configure your email settings in settings.py
. For development, Django
provides a convenient backend that writes emails to the console.
# settings.py
EMAIL_BACKEND = 'django.core.mail.backends.console.EmailBackend'
This setup is perfect for development as it allows you to see email contents directly in the terminal.
Step 2: Using SMTP for Development
If you need to test sending emails via SMTP, configure Django to use a real SMTP server. For instance, you can use Gmail's SMTP server:
# settings.py
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = 'smtp.gmail.com'
EMAIL_PORT = 587
EMAIL_USE_TLS = True
EMAIL_HOST_USER = 'your_email@gmail.com'
EMAIL_HOST_PASSWORD = 'your_email_password'
Make sure to use environment variables or Django's decouple
package to manage
sensitive information securely.
Sending Emails in Django
Step 3: Create a View to Send Email
Create a simple view that sends an email. For demonstration, let's add a view in
views.py
:
# views.py
from django.core.mail import send_mail
from django.http import HttpResponse
def send_test_email(request):
send_mail(
'Subject - Test Email',
'Here is the message body.',
'from@example.com',
['to@example.com'],
fail_silently=False,
)
return HttpResponse("Email sent successfully!")
Step 4: Map the View to a URL
Add a URL pattern for the email sending view in urls.py
:
# urls.py
from django.urls import path
from .views import send_test_email
urlpatterns = [
path('send-email/', send_test_email, name='send_email'),
]
Step 5: Testing the Email
Run your Django development server:
python manage.py runserver
Navigate to http://localhost:8000/send-email/
in your browser. If configured
correctly, you should see a message indicating that the email was sent, and the
email contents should appear in your console (or be sent via the SMTP server if
configured).
Conclusion
Configuring Django mailer in your development environment is straightforward with Django's built-in email backends. Using the console backend is excellent for local development, while configuring an SMTP backend allows for more comprehensive testing. Ensure sensitive information is securely managed using environment variables.
By following these steps, you can effectively test and implement email functionality in your Django application during development.