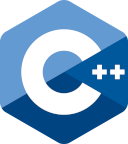
Makefiles are powerful tools for automating the build process of C++ projects. While they can seem intimidating at first, creating a basic Makefile doesn't have to be a daunting task. In this blog post, we'll walk through a straightforward example of a Makefile for a C++ project. By the end of this guide, you'll have a solid understanding of how Makefiles work and how they can simplify the compilation process.
Why Use a Makefile?
Before diving into our example, let's briefly discuss why Makefiles are essential for C++ development:
- Automation: Makefiles automate the build process, ensuring that only modified source files are recompiled. This saves time and resources.
- Dependency Management: Makefiles handle dependencies, so you don't have to worry about compiling files in the correct order.
- Portability: Makefiles work on various platforms and are not tied to a specific development environment or IDE.
The Anatomy of a Simple Makefile
Here's a basic Makefile for a C++ project:
# Compiler and Compiler Flags
CC = g++
CFLAGS = -std=c++11 -Wall
# Source and Object Files
SRC = main.cpp foo.cpp bar.cpp
OBJ = $(SRC:.cpp=.o)
# Executable Name
EXECUTABLE = myapp
# Default Target
all: $(EXECUTABLE)
# Compile Source Files into Object Files
%.o: %.cpp
$(CC) $(CFLAGS) -c $< -o $@
# Link Object Files to Create Executable
$(EXECUTABLE): $(OBJ)
$(CC) $(OBJ) -o $@
# Clean Build Artifacts
clean:
rm -f $(OBJ) $(EXECUTABLE)
Now, let's break down the key components of this Makefile:
- Compiler and Compiler Flags:
CC
specifies the C++ compiler (in this case,g++
).CFLAGS
defines compiler flags, such as-std=c++11
for C++11 compatibility and-Wall
for enabling warnings.
- Source and Object Files:
SRC
lists all source files in your project (e.g.,main.cpp
,foo.cpp
,bar.cpp
).OBJ
is generated by replacing the.cpp
extension inSRC
with.o
. It represents the object files.
- Executable Name:
EXECUTABLE
specifies the name of the output executable (e.g.,myapp
).
- Default Target:
- The
all
target is the default target that gets executed when you runmake
without specifying a target.
- The
- Compile Source Files into Object Files:
- This rule tells Make how to compile individual
.cpp
files into.o
(object) files.
- This rule tells Make how to compile individual
- Link Object Files to Create Executable:
- This rule specifies how to link object files to create the final executable.
- Clean Build Artifacts:
- The
clean
target is used to remove all generated object files and the executable.
- The
Using the Makefile
Here's how you can use the Makefile to build your C++ project:
- Create a directory with the same structure as the Makefile (source files in the same directory).
- Place the Makefile in the project directory.
- Open a terminal and navigate to your project directory.
- To build your project, simply run
make
. - To clean up generated files, run
make clean
.
Conclusion
Makefiles are essential tools for automating the build process in C++ projects. While they may seem complex at first, creating a simple Makefile like the one in this example can significantly streamline your development workflow. As your project grows, you can extend your Makefile to handle more complex scenarios and dependencies. Happy coding!
Want to read more? Check out "Managing Projects with GNU Make" by Robert Mecklenburg