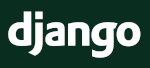
When developing Django applications, you often need to filter and display query results dynamically within your templates. This can involve anything from simple lookups to complex filtering based on multiple conditions. This article provides an in-depth guide on various methods for filtering queries directly within Django templates.
Understanding the Basics
Django's template system is designed to be simple and to limit logic in templates. Therefore, most filtering logic should ideally be handled in views or model methods. However, there are scenarios where you might need to filter querysets within templates using template tags and filters.
Filtering in Views
Before diving into template filtering, it's important to know how to filter querysets in views, as this is the recommended practice.
Example
# views.py
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.filter(is_available=True)
return render(request, 'product_list.html', {'products': products})
In this example, we filter the Product
model to get only available products and
pass this filtered queryset to the template.
Using Template Filters
Built-in Template Filters
Django provides built-in template filters to manipulate data. For instance, the
|length
filter returns the length of a list.
<!-- product_list.html -->
{% if products|length > 0 %}
<p>We have {{ products|length }} products available.</p>
{% else %}
<p>No products available.</p>
{% endif %}
Custom Template Filters
For more advanced filtering, you can create custom template filters.
Step 1: Create a Custom Filter
Create a templatetags
directory in your app and add a Python file (e.g.,
custom_filters.py
).
# templatetags/custom_filters.py
from django import template
register = template.Library()
@register.filter
def available_products(products):
return products.filter(is_available=True)
Step 2: Load and Use the Custom Filter in Template
{% load custom_filters %}
<ul>
{% for product in products|available_products %}
<li>{{ product.name }}</li>
{% endfor %}
</ul>
In this example, the available_products
filter is applied to the products
queryset to show only available products.
Using Template Tags
Custom template tags offer more flexibility for complex operations.
Simple Custom Template Tag
Step 1: Define a Simple Tag
Create a custom template tag that filters products by category.
# templatetags/custom_tags.py
from django import template
from ..models import Product
register = template.Library()
@register.simple_tag
def products_by_category(category):
return Product.objects.filter(category=category, is_available=True)
Step 2: Use the Custom Tag in Template
{% load custom_tags %}
<h2>Electronics</h2>
<ul>
{% products_by_category 'electronics' as electronics %}
{% for product in electronics %}
<li>{{ product.name }}</li>
{% endfor %}
</ul>
Inclusion Tags
Inclusion tags render a template fragment with a context.
Step 1: Define an Inclusion Tag
# templatetags/custom_tags.py
@register.inclusion_tag('product_list.html')
def show_products(category):
products = Product.objects.filter(category=category, is_available=True)
return {'products': products}
Step 2: Create a Template Fragment
<!-- templates/product_list.html -->
<ul>
{% for product in products %}
<li>{{ product.name }}</li>
{% endfor %}
</ul>
Step 3: Use the Inclusion Tag in Template
{% load custom_tags %}
<h2>Electronics</h2>
{% show_products 'electronics' %}
Best Practices
- Filter in Views or Models: Prefer filtering in views or model methods to keep templates simple.
- Use Template Filters Sparingly: Use built-in or custom template filters for minor data manipulations.
- Employ Template Tags for Complex Logic: Use custom template tags for more complex filtering or when you need to reuse the logic.
Conclusion
Filtering queries directly in Django templates can be powerful but should be used judiciously. The recommended approach is to handle most filtering logic in views or model methods. For cases where template filtering is necessary, Django’s template system offers robust tools like custom filters and tags to help you manage your data efficiently. By following best practices and leveraging these tools, you can create dynamic and responsive Django applications.