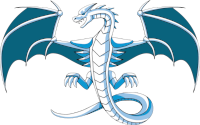
LLVM, which stands for "Low Level Virtual Machine," is not just a compiler infrastructure; it's a powerful ecosystem for developing compilers, code analysis tools, and more. Whether you're a seasoned developer looking to explore the inner workings of LLVM or a newcomer intrigued by its capabilities, this expert-level guide will take you through the journey of getting started with LLVM and unlocking its full potential.
Prerequisites
Before diving into LLVM, make sure you have the following prerequisites:
- C/C++ Knowledge: A solid understanding of C and C++ is essential, as LLVM is primarily written in these languages.
- Development Environment: Set up a development environment with a C/C++ compiler (e.g., Clang or GCC), CMake, Git, and Python.
- Operating System: LLVM is compatible with various operating systems, including Linux, macOS, and Windows.
- Build Tools: Ensure you have tools like
make
andninja
for building LLVM.
Step 1: Clone the LLVM Repository
Begin by cloning the LLVM repository from GitHub. Open your terminal and run:
git clone https://github.com/llvm/llvm-project.git
This command will create a directory named "llvm-project" containing the LLVM source code and related projects.
Step 2: Configure the Build
Navigate to the "llvm-project" directory and create a "build" directory within it:
cd llvm-project
mkdir build
cd build
Now, configure the build using CMake, specifying your preferred build system (e.g., Ninja):
cmake -G "Ninja" -DLLVM_ENABLE_PROJECTS="clang;lld" ../llvm
This command configures the build with Clang (the C/C++ frontend) and LLD (the linker) enabled.
Step 3: Build LLVM
You can now initiate the build process:
ninja
Building LLVM may take a significant amount of time, especially on slower systems or when building all LLVM components. Be patient and let the build process complete.
Step 4: Test LLVM
After the build is successful, it's crucial to run LLVM's test suite to ensure its correctness:
ninja check-all
Running the test suite helps verify that LLVM is functioning correctly, and it's a critical step to confirm the integrity of your build.
Step 5: Explore LLVM
Now that you have LLVM up and running, it's time to explore its capabilities. Here are some advanced avenues to consider:
- Write a Custom Compiler: Dive deep into LLVM's APIs to build a custom compiler for your programming language of choice. LLVM IR serves as an intermediary language for code generation.
- Optimize Code: Experiment with LLVM's powerful optimization passes to understand how code transformations work and improve code performance.
- Develop Code Analysis Tools: Leverage LLVM's rich analysis infrastructure to create code analysis tools like static analyzers, profilers, or security scanners.
- Extend LLVM: Explore LLVM's extensibility by developing custom optimization passes, target-specific backends, or even new LLVM frontends for different languages.
- Contribute to LLVM: Join the LLVM community by contributing code, bug reports, or documentation updates on GitHub. Engaging with the community can be an enriching experience.
- Read the Documentation: LLVM provides comprehensive documentation that covers its components, APIs, and development guidelines. It's an invaluable resource for in-depth learning.
- Advanced Topics: Consider exploring advanced topics like Just-In-Time (JIT) compilation, custom code generation, or using LLVM in embedded systems.
Conclusion
Getting started with LLVM is just the beginning of an exciting journey into the world of compilers, code analysis, and optimization. As you delve deeper, you'll discover the immense power and flexibility that LLVM offers to developers and researchers alike. Whether you're building a new language, optimizing code, or contributing to the LLVM project, you're embarking on a path that leads to a deeper understanding of the intricacies of modern software development. Happy coding!