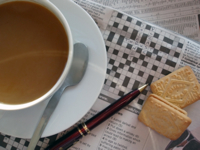
In today's complex network environments, monitoring traffic efficiently is crucial for maintaining performance and security. Tools like Cisco NBAR (Network-Based Application Recognition) and MRTG (Multi Router Traffic Grapher) can provide valuable insights into network usage. In this article, we'll discuss how to use a Perl script with MRTG to collect and graph traffic data from Cisco NBAR Protocol Discovery. We'll also update the OIDs (Object Identifiers) used in the script to ensure you're working with the most current standards.
The Power of Cisco NBAR and MRTG
Cisco NBAR is a powerful tool for classifying and monitoring network traffic. By recognizing and categorizing application-level protocols, NBAR helps network administrators understand what types of traffic are traversing their networks. MRTG is a widely-used tool for graphing network data, making it easier to visualize traffic patterns and performance metrics.
Combining these tools allows for detailed traffic analysis and visualization, which is essential for effective network management.
Writing a Perl Script for MRTG
To collect and graph traffic data from Cisco NBAR using MRTG, we need a Perl script that queries the relevant SNMP OIDs. Here's an updated version of the script:
#!/usr/bin/perl
use strict;
use warnings;
use Net::SNMP;
my $hostname = 'your.router.hostname';
my $community = 'public';
# Define OIDs for Cisco NBAR Protocol Discovery
my $oid_protocol_name = '.1.3.6.1.4.1.9.9.244.1.8.1.1.2';
my $oid_in_bytes = '.1.3.6.1.4.1.9.9.244.1.2.1.1.9';
my $oid_out_bytes = '.1.3.6.1.4.1.9.9.244.1.2.1.1.10';
# Create a SNMP session
my ($session, $error) = Net::SNMP->session(
-hostname => $hostname,
-community => $community,
-version => 'snmpv2c'
);
if (!defined $session) {
printf "ERROR: %s.\n", $error;
exit 1;
}
# Fetch protocol names
my $result = $session->get_table(-baseoid => $oid_protocol_name);
if (!defined $result) {
printf "ERROR: %s.\n", $session->error;
$session->close;
exit 1;
}
# Print protocol names and their OIDs
foreach my $oid (keys %{$result}) {
printf "%s => %s\n", $oid, $result->{$oid};
}
$session->close;
This script initializes a session with the SNMP server and queries the protocol names that NBAR can recognize. You can expand this script to fetch input and output bytes for specific protocols and interfaces by appending the necessary indices.
Understanding the OIDs
To work with Cisco NBAR Protocol Discovery MIB, you need to understand the relevant OIDs:
cnpdSupportedProtocolsName
OID: .1.3.6.1.4.1.9.9.244.1.8.1.1.2
This OID provides a list of protocols that NBAR can recognize. Since the index of a particular protocol might change, it's crucial to fetch the index from this table and reference it in subsequent queries.
cnpdAllStatsHCInBytes
OID: .1.3.6.1.4.1.9.9.244.1.2.1.1.9
This OID represents a table of input bytes sorted by interface and protocol. To
find the data for a specific protocol on a specific interface, append the
interface index followed by the protocol index (from
cnpdSupportedProtocolsName
).
cnpdAllStatsHCOutBytes
OID: .1.3.6.1.4.1.9.9.244.1.2.1.1.10
Similar to cnpdAllStatsHCInBytes
, this OID provides a table of output bytes
sorted by interface and protocol. Use it in the same manner by appending the
interface and protocol indices.
Using High-Capacity Counters
The byte counters listed above are "high capacity" 64-bit byte counters. It is recommended to use these counters whenever possible, especially for interfaces faster than Fast Ethernet. This ensures accurate monitoring over high-speed connections.
Conclusion
Monitoring network traffic using Cisco NBAR and MRTG provides valuable insights into your network's performance and security. By leveraging the power of Perl scripting and SNMP, you can create custom monitoring solutions tailored to your organization's needs.
For more detailed tutorials and updates on modern network management practices, stay tuned to our blog at slaptijack.com. If you have any questions or need further assistance, feel free to leave a comment or reach out. And remember, in the world of networking, proactive monitoring is key to maintaining a secure and efficient infrastructure. Happy monitoring!