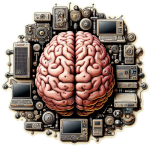
Predictive maintenance is transforming the way industries manage and maintain their assets. By predicting failures before they occur, companies can avoid unexpected downtimes, reduce maintenance costs, and extend the lifespan of their equipment. Leveraging machine learning for predictive maintenance is at the forefront of this transformation, providing robust and accurate predictions that help in proactive decision-making. In this article, we will explore how to leverage machine learning for predictive maintenance, from data collection and preprocessing to deploying predictive models.
Understanding Predictive Maintenance
Predictive maintenance involves using data analysis tools and techniques to detect anomalies and predict equipment failures before they occur. This approach allows maintenance to be performed just in time, rather than at fixed intervals or after a failure has occurred.
Benefits of Predictive Maintenance
- Reduced Downtime: By predicting failures before they happen, companies can schedule maintenance during non-peak times, minimizing disruptions.
- Cost Savings: Preventing unexpected failures reduces repair costs and extends equipment lifespan.
- Improved Safety: Predicting and preventing failures helps avoid accidents and ensures the safety of workers.
Data Collection and Preprocessing
The foundation of any machine learning model is data. For predictive maintenance, data typically comes from sensors monitoring various parameters of the equipment, historical maintenance records, and environmental data.
Types of Data Needed
- Sensor Data: Temperature, pressure, vibration, and other sensor readings provide real-time insights into the equipment's condition.
- Operational Data: Information on how the equipment is used, such as run-time hours and load conditions.
- Historical Maintenance Data: Records of past failures, repairs, and maintenance activities.
- Environmental Data: Conditions like humidity, temperature, and location that can affect equipment performance.
Data Preprocessing
Raw data needs to be cleaned and preprocessed to be useful for machine learning models. This involves:
- Data Cleaning: Removing noise, handling missing values, and correcting errors.
- Feature Engineering: Creating new features from the raw data that better capture the underlying patterns.
- Normalization: Scaling the data to ensure that all features contribute equally to the model.
Building Predictive Models
Choosing the right machine learning algorithm is crucial for building accurate predictive maintenance models. Common algorithms used include regression models, classification models, and time-series analysis.
Choosing the Right Algorithms
- Regression Models: Used to predict the remaining useful life (RUL) of equipment.
- Classification Models: Used to classify the equipment's state as healthy or faulty.
- Time-Series Analysis: Used to analyze sensor data over time to detect trends and patterns.
Training and Validating Models
- Training: The model is trained using historical data to learn the relationship between input features and the target variable (e.g., equipment failure).
- Validation: The model is validated using a separate dataset to ensure it generalizes well to new data.
- Evaluation Metrics: Common metrics include Mean Absolute Error (MAE) for regression models and F1 Score for classification models.
Example Using Python and Scikit-Learn
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# Load dataset
data = pd.read_csv('sensor_data.csv')
# Preprocess data
data.fillna(data.mean(), inplace=True)
features = data.drop('failure', axis=1)
target = data['failure']
# Split data
X_train, X_test, y_train, y_test = train_test_split(
features,
target,
test_size=0.2,
random_state=42,
)
# Train model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Predict and evaluate
y_pred = model.predict(X_test)
print(classification_report(y_test, y_pred))
Deploying Machine Learning Models
Once the model is trained and validated, it needs to be deployed to make predictions in real-time.
Using Frameworks
- TensorFlow and Keras: For deep learning models.
- Scikit-Learn: For traditional machine learning models.
Integrating Models with Existing Systems
- REST API: Expose the model as a REST API using frameworks like Flask or FastAPI.
- Streaming Data: Use platforms like Apache Kafka to handle real-time data streams and make predictions on-the-fly.
Example Using Flask
from flask import Flask, request, jsonify
import joblib
app = Flask(__name__)
model = joblib.load('predictive_model.pkl')
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json(force=True)
prediction = model.predict([data['features']])
return jsonify({'prediction': prediction[0]})
if __name__ == '__main__':
app.run(debug=True)
Case Studies
1. General Electric
General Electric (GE) uses predictive maintenance to monitor and maintain its fleet of jet engines. By analyzing sensor data in real-time, GE can predict engine failures before they occur, reducing downtime and maintenance costs.
2. Siemens
Siemens leverages machine learning for predictive maintenance in its manufacturing plants. By predicting equipment failures, Siemens can perform maintenance proactively, increasing the efficiency and reliability of its operations.
Best Practices and Lessons Learned
- Data Quality: Ensure high-quality data collection and preprocessing.
- Continuous Monitoring: Regularly update and retrain models with new data.
- Collaboration: Work closely with domain experts to understand the equipment and its failure modes.
Conclusion
Leveraging machine learning for predictive maintenance provides significant benefits, including reduced downtime, cost savings, and improved safety. By following a systematic approach from data collection and preprocessing to model deployment, companies can harness the power of predictive maintenance to optimize their operations.
For more in-depth tutorials and insights into modern software development practices, stay tuned to our blog at https://slaptijack.com. If you have any questions or need further assistance, feel free to reach out. Happy coding and happy maintaining!