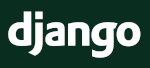
In Django, ForeignKey
is a powerful tool for creating relationships between
models. There are scenarios where you may want to limit the choices for a
ForeignKey
field to a specific subset of users, such as staff members. This
article provides a comprehensive guide on how to limit a ForeignKey
to staff
users in Django, covering different approaches and best practices.
Prerequisites
Before you begin, ensure you have the following:
- Python 3.6 or higher
- Django installed (version 3.0 or higher)
- Basic understanding of Django models and admin interface
Understanding ForeignKey in Django
A ForeignKey
in Django creates a many-to-one relationship between two models.
It is represented as a database column that references the primary key of another
table.
Example of a Basic ForeignKey
Here is an example of a basic ForeignKey
relationship:
from django.db import models
from django.contrib.auth.models import User
class Project(models.Model):
name = models.CharField(max_length=100)
owner = models.ForeignKey(User, on_delete=models.CASCADE)
def __str__(self):
return self.name
In this example, each Project
is associated with a User
through the owner
field.
Limiting ForeignKey Choices to Staff Users
To limit the choices for a ForeignKey
to staff users, you can use several
approaches. We will cover the following methods:
- Customizing the Model Field
- Overriding the Form Field in Admin
- Using a Custom Manager
- Implementing a Custom Widget
Method 1: Customizing the Model Field
One straightforward approach is to customize the form field that corresponds to
the ForeignKey
in the model's admin form.
Step-by-Step Guide
-
Create the Project Model:
from django.db import models from django.contrib.auth.models import User class Project(models.Model): name = models.CharField(max_length=100) owner = models.ForeignKey( User, on_delete=models.CASCADE, limit_choices_to={'is_staff': True} ) def __str__(self): return self.name
The
limit_choices_to
parameter allows you to specify a dictionary of conditions that the referenced model's objects must meet. -
Register the Model in Admin:
from django.contrib import admin from .models import Project @admin.register(Project) class ProjectAdmin(admin.ModelAdmin): list_display = ('name', 'owner')
This method works well for limiting choices in the admin interface and forms generated by Django, but it has some limitations in terms of flexibility.
Method 2: Overriding the Form Field in Admin
For more control over the ForeignKey
choices, you can override the form field
in the admin class.
Step-by-Step Guide
-
Create a Custom Form:
from django import forms from django.contrib.auth.models import User from .models import Project class ProjectForm(forms.ModelForm): class Meta: model = Project fields = '__all__' def __init__(self, *args, **kwargs): super().__init__(*args, **kwargs) self.fields['owner'].queryset = User.objects.filter(is_staff=True)
-
Register the Model with the Custom Form:
from django.contrib import admin from .models import Project from .forms import ProjectForm @admin.register(Project) class ProjectAdmin(admin.ModelAdmin): form = ProjectForm list_display = ('name', 'owner')
This approach provides greater flexibility as you can add more complex filtering logic if needed.
Method 3: Using a Custom Manager
Another approach is to use a custom manager to handle the filtering logic.
Step-by-Step Guide
-
Create a Custom Manager:
from django.contrib.auth.models import UserManager class StaffUserManager(UserManager): def get_queryset(self): return super().get_queryset().filter(is_staff=True)
-
Create a Proxy Model:
from django.contrib.auth.models import User class StaffUser(User): objects = StaffUserManager() class Meta: proxy = True
-
Update the Project Model:
from django.db import models class Project(models.Model): name = models.CharField(max_length=100) owner = models.ForeignKey(StaffUser, on_delete=models.CASCADE) def __str__(self): return self.name
Method 4: Implementing a Custom Widget
For even more control, you can create a custom form widget.
Step-by-Step Guide
-
Create a Custom Widget:
from django import forms from django.contrib.auth.models import User class StaffUserWidget(forms.Select): def __init__(self, attrs=None): super().__init__(attrs) self.choices = [ (user.id, user.username) for user in User.objects.filter(is_staff=True) ]
-
Create a Custom Form:
class ProjectForm(forms.ModelForm): class Meta: model = Project fields = '__all__' widgets = { 'owner': StaffUserWidget(), }
-
Register the Model with the Custom Form:
from django.contrib import admin from .models import Project from .forms import ProjectForm @admin.register(Project) class ProjectAdmin(admin.ModelAdmin): form = ProjectForm list_display = ('name', 'owner')
Conclusion
Limiting a ForeignKey
to staff users in Django can be achieved through several
methods, each with its own advantages and use cases. Whether you choose to
customize the model field, override the form field in admin, use a custom
manager, or implement a custom widget, Django provides the flexibility to tailor
the solution to your needs. By following the steps outlined in this article, you
can effectively manage ForeignKey
relationships and ensure that only staff
users are selectable in your Django applications.