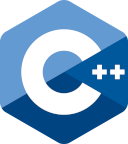
Structuring a C++ project effectively is crucial for code maintainability, collaboration, and scalability. A well-organized project enhances productivity and makes it easier to maintain code over time. In this article, we'll explore best practices and guidelines for structuring a C++ project, helping you create a solid foundation for your software development.
Directory Structure
Organizing your project files and folders is key:
project_name/
|-- src/
| |-- main.cpp
| |-- module1/
| | |-- module1.cpp
| | |-- module1.h
| |-- module2/
| | |-- module2.cpp
| | |-- module2.h
|-- include/
| |-- project_name/
| | |-- module1.h
| | |-- module2.h
|-- lib/
|-- tests/
| |-- unit_tests.cpp
|-- build/
|-- doc/
|-- CMakeLists.txt
|-- README.md
- src: Contains source code files, including the main application entry point and various modules.
- include: Holds header files (
.h
or.hpp
) defining module interfaces. - lib: For third-party libraries or external dependencies.
- tests: Contains unit tests.
- build: Directory for build artifacts.
- doc: Storage for documentation files.
- CMakeLists.txt: The CMake configuration file.
- README.md: Markdown documentation with project instructions.
Build System
Choose CMake for managing C++ project builds. It generates platform-specific build files and offers portability and flexibility.
Source Code Organization
- Divide code into modules or components in separate directories.
- Implement the "single responsibility principle."
- Use namespaces to prevent naming conflicts.
- Minimize global variables and functions.
Header Files
- Use include guards or
#pragma once
to prevent multiple inclusions. - Include only what's necessary in header files.
- Use forward declarations when possible.
Dependency Management
- Utilize package managers like
vcpkg
or system package managers. - Document external dependencies and provide installation instructions.
Testing
- Use a unit testing framework like Google Test or Catch2.
- Create a separate directory for unit tests.
Documentation
- Employ a documentation tool like Doxygen.
- Include high-level project documentation.
Version Control
- Use Git for tracking changes and collaborating.
- Follow version control best practices.
Continuous Integration (CI)
- Set up CI/CD pipelines to automate builds, testing, and deployment.
- Platforms like Jenkins, Travis CI, or GitHub Actions can simplify this process.
Conclusion
A well-structured C++ project enhances code quality, collaboration, and scalability. Whether for personal or team projects, following these guidelines will set you on the path to success.