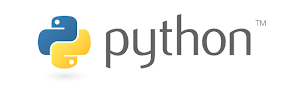
Occasionally, you may run across a date in a format that can't be easily loaded into a Python date object. For example, I recently had a situation where a file I was parsing was full of dates in this format: 7/7/2008.
The obvious thing to do here is to split the date into it's component parts and then create the date object:
from datetime import date
month, day, year = "7/7/2008".split("/")
expires_on = date(int(year), int(month), int(day))
This works, but all those int()
's are pretty ugly. Python's map()
function
can fix this up. The map()
function was designed to apply a function to every
item in an iterable. In this case, we want to apply int()
to each part of the
string before assigning to the variables. That way we've got the integers that
date()
requires:
from datetime import date
month, day, year = map(int, "7/7/2008".split("/"))
expires_on = date(year, month, day)