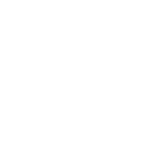
Systems programming has traditionally been dominated by languages like C and C++. However, in recent years, a new contender has emerged that promises to bring safety, speed, and modern features to the forefront of systems-level development: Rust. Rust, developed by Mozilla, has quickly gained popularity for its focus on memory safety, concurrency, and performance without sacrificing control over low-level details. In this article, we will explore why Rust is becoming the go-to language for systems programming, its key features, and its growing adoption in the industry.
What is Rust?
Rust is a systems programming language designed to be fast, memory-safe, and concurrent. It was initially developed by Mozilla Research and has since grown into a community-driven project. Rust aims to provide the performance and control of languages like C and C++ while eliminating common programming errors that lead to security vulnerabilities and unstable code.
Key Features of Rust
- Memory Safety: Rust’s ownership system ensures that memory safety is enforced at compile time, preventing common bugs such as null pointer dereferencing, buffer overflows, and use-after-free errors. This system eliminates the need for a garbage collector, allowing for predictable performance.
- Zero-Cost Abstractions: Rust provides high-level abstractions without compromising performance. Features like pattern matching, iterators, and algebraic data types (enums) are designed to be as efficient as handwritten low-level code.
- Concurrency: Rust's type system ensures safe concurrency by preventing data races at compile time. The language's ownership model allows developers to write concurrent programs without worrying about traditional concurrency issues.
- Performance: Rust's performance is on par with C and C++. It compiles to native code and can be used for low-level programming tasks where performance is critical.
- Modern Tooling: Rust comes with a robust package manager and build system called Cargo, integrated documentation, and comprehensive testing frameworks. The language also has excellent support for IDEs and editors.
Why Rust is the Future of Systems Programming
1. Memory Safety
Memory safety is one of the most significant challenges in systems programming. Languages like C and C++ give developers full control over memory management, which can lead to severe bugs and security vulnerabilities if not handled correctly. Rust addresses this by enforcing strict ownership and borrowing rules, ensuring that memory safety is guaranteed at compile time. This reduces the risk of bugs and makes systems programming more accessible and secure.
2. Concurrency Without Data Races
Concurrency is essential for modern software development, especially in systems programming, where performance and efficiency are critical. Rust’s ownership model and type system ensure that data races are caught at compile time, making it easier to write safe concurrent code. This is a significant advantage over traditional languages, where concurrency issues often lead to hard-to-diagnose bugs.
3. High Performance
Rust’s performance is comparable to C and C++, making it suitable for systems-level programming where speed is crucial. The language's design allows for fine-grained control over hardware resources, ensuring that developers can write highly efficient code. Additionally, Rust's zero-cost abstractions mean that high-level constructs do not incur runtime overhead, providing both safety and performance.
4. Strong Ecosystem and Community
Rust has a rapidly growing ecosystem and a strong, supportive community. The language's package manager, Cargo, simplifies dependency management and project setup. The Rust community actively contributes to the ecosystem, creating libraries and tools that make development more straightforward and more productive. The language's comprehensive documentation and community-driven support also make it easier for new developers to get started with Rust.
5. Industry Adoption
Rust is increasingly being adopted by major companies for systems programming. Notable examples include:
- Mozilla: Rust was initially developed by Mozilla for the Servo web browser engine, designed to provide safety and concurrency.
- Microsoft: Microsoft has explored using Rust for secure and efficient system components, particularly in the context of operating systems.
- Amazon Web Services (AWS): AWS uses Rust for performance-critical services like the Firecracker microVM.
- Facebook: Facebook has adopted Rust for building secure and reliable infrastructure tools.
Key Rust Concepts
Ownership and Borrowing
Rust’s ownership model is its most distinctive feature. Here’s a brief overview:
- Ownership: Each value in Rust has a variable that owns it. When the owning variable goes out of scope, the value is dropped, and memory is freed.
- Borrowing: References to values can be borrowed, allowing multiple parts of a program to access the data without transferring ownership. Borrowing can be mutable or immutable, but mutable references are exclusive.
- Lifetimes: Rust uses lifetimes to track how long references are valid, ensuring that references do not outlive the data they point to.
Pattern Matching
Rust’s pattern matching is powerful and expressive, allowing developers to match complex data structures and perform different actions based on their shape.
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
ChangeColor(i32, i32, i32),
}
fn process_message(msg: Message) {
match msg {
Message::Quit => println!("Quit message"),
Message::Move { x, y } => println!("Move to ({}, {})", x, y),
Message::Write(text) => println!("Write message: {}", text),
Message::ChangeColor(r, g, b) => println!(
"Change color to ({}, {}, {})", r, g, b
),
}
}
Error Handling
Rust uses the Result
and Option
types for error handling, providing a robust
way to manage potential errors without relying on exceptions.
fn divide(dividend: f64, divisor: f64) -> Result<f64, String> {
if divisor == 0.0 {
Err(String::from("Cannot divide by zero"))
} else {
Ok(dividend / divisor)
}
}
fn main() {
match divide(4.0, 2.0) {
Ok(result) => println!("Result: {}", result),
Err(e) => println!("Error: {}", e),
}
}
Getting Started with Rust
Installation
Rust can be installed using the Rustup installer, which manages Rust versions and associated tools.
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
After installation, you can verify it with:
rustc --version
Creating a New Project
Rust projects are managed with Cargo. To create a new project:
cargo new hello_rust
cd hello_rust
Writing and Running Code
Rust code is written in .rs
files. The main.rs
file is the entry point for
most applications.
fn main() {
println!("Hello, Rust!");
}
To build and run the project:
cargo build
cargo run
Using External Crates
Cargo makes it easy to include external libraries (crates). Add dependencies to
Cargo.toml
.
[dependencies]
rand = "0.8"
Use the crate in your code:
use rand::Rng;
fn main() {
let mut rng = rand::thread_rng();
let n: u32 = rng.gen();
println!("Random number: {}", n);
}
Conclusion
Rust is rapidly becoming the language of choice for systems programming due to its focus on memory safety, concurrency, and performance. Its modern features, strong ecosystem, and growing industry adoption make it an excellent choice for developers looking to build reliable, efficient, and secure systems. By understanding and leveraging Rust’s unique capabilities, software engineers can write code that not only performs well but also avoids many common pitfalls associated with systems programming. As the tech industry continues to evolve, Rust is well-positioned to play a crucial role in shaping the future of software development.