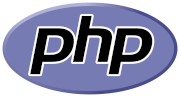
Storing PHP sessions effectively is crucial for managing user data and maintaining session persistence in web applications. PHP provides multiple options for session storage, and the choice depends on factors like performance, scalability, and the specific needs of your application. Here are some strategies for storing PHP sessions:
-
File-based Session Storage:
PHP's default session handling mechanism involves storing session data on the server's filesystem. This is a simple and widely used method. To configure PHP to use file-based storage, ensure the following settings in your
php.ini
file:session.save_handler = files session.save_path = /path/to/session/files
Pros:
- Easy to set up.
- Works well for small to medium-sized applications.
- No additional dependencies.Cons:
- May not scale well for large applications with high traffic due to file I/O.
- Potential security risks if session files are accessible by unauthorized users. -
Database Session Storage:
Storing sessions in a relational database is a scalable and secure approach. Create a database table to store session data, and configure PHP to use this storage method:
session.save_handler = user session.save_path = "user"
Then, implement custom session handling functions in your PHP code to read and write session data to the database.
Pros:
- Scalable and suitable for large applications.
- Data can be easily secured using database access controls.
- Allows for better control over session data.Cons:
- Requires additional setup and database access.
- Slightly slower than file-based storage due to database interactions. -
In-memory Session Storage:
Storing sessions in memory, such as using Memcached or Redis, is an excellent choice for optimizing session access speed. You'll need to install and configure the chosen in-memory data store and then configure PHP accordingly:
session.save_handler = memcached session.save_path = "tcp://localhost:11211"
Pros:
- Fast and efficient for session access.
- Suitable for high-traffic websites.
- Allows for distributed session storage for load balancing.Cons:
- Requires additional infrastructure (e.g., Memcached or Redis) and maintenance.
- Data may not be persisted in case of server restarts. -
Custom Session Handlers:
If none of the built-in storage options fit your requirements, you can create a custom session handler. Implementing a custom session handler allows you to store session data wherever you need, such as in a cloud-based database or a NoSQL store.
To create a custom session handler, you must define your own functions for session management using
session_set_save_handler
. Here's a simplified example:// Define custom session functions function custom_session_open($savePath, $sessionName) { /* ... */ } function custom_session_close() { /* ... */ } function custom_session_read($sessionId) { /* ... */ } function custom_session_write($sessionId, $data) { /* ... */ } function custom_session_destroy($sessionId) { /* ... */ } function custom_session_gc($maxLifetime) { /* ... */ } // Register the custom session handler session_set_save_handler( 'custom_session_open', 'custom_session_close', 'custom_session_read', 'custom_session_write', 'custom_session_destroy', 'custom_session_gc' ); // Start the session session_start();
Pros:
- Provides the flexibility to store sessions in any manner.
- Ideal for unconventional storage needs.Cons:
- Requires a deeper understanding of PHP's session management.
- Can be more complex to set up and maintain.
Choosing the right session storage strategy depends on your application's requirements and scalability needs. For small to medium-sized applications, file-based or database storage may suffice. In-memory storage using technologies like Memcached or Redis is excellent for high-performance applications. Custom session handlers offer the most flexibility but require a deeper understanding of PHP's session management. Make your choice based on your project's specific needs and constraints.