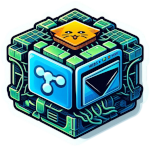
In the world of web development, real-time communication has become increasingly crucial. From live chat applications and online gaming to real-time data streaming and collaborative editing tools, the demand for instantaneous data exchange between clients and servers has surged. WebSockets, a powerful protocol introduced to address this need, enable full-duplex communication channels over a single, long-lived TCP connection. This article delves into what WebSockets are, how they work, and their practical applications.
What Are WebSockets?
Definition
WebSockets are a communication protocol providing full-duplex channels over a single TCP connection. Unlike traditional HTTP, which is request-response based and stateless, WebSockets allow for continuous, bidirectional communication between the client and server. This means that either party can send data to the other at any time, making it ideal for applications requiring real-time updates.
Origin and Standardization
The WebSocket protocol was standardized by the IETF as RFC 6455 in 2011. Its API was also standardized by the W3C. This standardization ensures broad compatibility and support across modern web browsers and server platforms.
How WebSockets Work
Handshake Process
The WebSocket connection begins with a handshake, which is essentially an HTTP request/response upgrade mechanism. Here's a simplified version of how it works:
-
Client Request: The client sends an HTTP request to the server with an
Upgrade
header, indicating the desire to establish a WebSocket connection.GET /chat HTTP/1.1 Host: server.example.com Upgrade: websocket Connection: Upgrade Sec-WebSocket-Key: dGhlIHNhbXBsZSBub25jZQ== Sec-WebSocket-Version: 13
-
Server Response: If the server supports WebSockets, it responds with an HTTP 101 status code, indicating that the protocol is switching to WebSockets.
HTTP/1.1 101 Switching Protocols Upgrade: websocket Connection: Upgrade Sec-WebSocket-Accept: s3pPLMBiTxaQ9kYGzzhZRbK+xOo=
Data Frames
Once the connection is established, data is exchanged in the form of frames. These frames can contain text, binary data, or control information.
- Text Frames: UTF-8 encoded text.
- Binary Frames: Arbitrary binary data.
- Control Frames: Special frames for controlling the connection (e.g., closing the connection, ping/pong for keep-alive).
Maintaining the Connection
WebSocket connections are persistent, meaning they stay open until explicitly closed by either the client or server. This persistence reduces the overhead of establishing a new connection for each data exchange, thus enhancing performance and reducing latency.
Practical Applications of WebSockets
Real-Time Chat Applications
WebSockets are ideal for building real-time chat applications. They allow for instant message exchange between users without the need to refresh the page or repeatedly poll the server.
const ws = new WebSocket('ws://example.com/chat');
ws.onmessage = (event) => {
const message = event.data;
console.log(`Received message: ${message}`);
};
ws.send('Hello, World!');
Online Gaming
In online multiplayer games, WebSockets facilitate real-time interaction between players by providing a low-latency communication channel.
Real-Time Data Feeds
Financial services, stock tickers, and news feeds can leverage WebSockets to push real-time updates to users, ensuring they have the most current information without delay.
Collaborative Editing
Applications like Google Docs use WebSockets to enable multiple users to edit a document simultaneously, with changes reflected in real-time across all clients.
Advantages of WebSockets
Low Latency
WebSockets significantly reduce latency compared to traditional HTTP, as the connection remains open, allowing for instantaneous data transmission.
Efficiency
By maintaining a persistent connection, WebSockets reduce the overhead associated with establishing and closing multiple HTTP connections.
Simplified Communication
The bidirectional nature of WebSockets simplifies the implementation of features that require real-time data exchange, as data can be sent and received at any time.
Security Considerations
While WebSockets offer numerous advantages, it's essential to consider security implications:
- Encryption: Use WSS (WebSocket Secure) to encrypt data in transit, ensuring privacy and integrity.
- Authentication: Implement robust authentication mechanisms to prevent unauthorized access.
- Rate Limiting: Protect against denial-of-service attacks by limiting the rate of incoming connections and messages.
Conclusion
WebSockets are a game-changer for real-time web applications, providing a robust and efficient means of continuous communication between clients and servers. Whether you're building chat applications, online games, real-time data feeds, or collaborative tools, understanding and leveraging WebSockets can significantly enhance your application's performance and user experience. For more in-depth tutorials and technical insights, keep exploring our blog. Happy coding!
For further reading and examples, check out the official WebSocket API documentation on MDN.