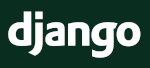
When working with Django projects, encountering errors during development and
deployment is common. One such error you might encounter when starting your
Django service using the ./manage.py runserver
command is:
Traceback (most recent call last):
File "./manage.py", line 11, in
execute_manager(settings)
File "/opt/local/Library/Frameworks/Python.framework/Versions/2.5/lib/python2.5/site-packages/django/core/management/__init__.py", line 436, in execute_manager
setup_environ(settings_mod)
File "/opt/local/Library/Frameworks/Python.framework/Versions/2.5/lib/python2.5/site-packages/django/core/management/__init__.py", line 419, in setup_environ
project_module = import_module(project_name)
File "/opt/local/Library/Frameworks/Python.framework/Versions/2.5/lib/python2.5/site-packages/django/utils/importlib.py", line 35, in import_module
__import__(name)
ImportError: No module named django.slaptijack.com
This article provides an in-depth analysis of the problem and detailed steps to resolve it.
Understanding the Error
The error message indicates that Django is unable to import the module
django.slaptijack.com
. This problem arises when the module name specified in
the settings is incorrect or the directory structure does not align with what
Django expects.
Breakdown of the Error
- Traceback Analysis: The error traceback shows the sequence of calls
leading to the error:
- The
./manage.py
script is executed. execute_manager(settings)
is called frommanage.py
.setup_environ(settings_mod)
is called withinexecute_manager
.import_module(project_name)
fails, raising anImportError
.
- The
- Core Issue: The
ImportError
indicates that Python cannot find the module nameddjango.slaptijack.com
. This issue generally results from:- Incorrect
DJANGO_SETTINGS_MODULE
environment variable. - Misconfigured project structure.
- Typographical errors in the module name.
- Incorrect
Steps to Resolve the Issue
1. Verify the Project Structure
Ensure that your project structure follows the standard Django layout. A typical Django project structure looks like this:
django.slaptijack.com/
manage.py
django/
slaptijack/
com/
__init__.py
settings.py
urls.py
wsgi.py
__init__.py
2. Check the DJANGO_SETTINGS_MODULE Environment Variable
The DJANGO_SETTINGS_MODULE
environment variable should point to the correct
settings module. It is usually set in the manage.py
file:
import os
import sys
if __name__ == "__main__":
os.environ.setdefault(
"DJANGO_SETTINGS_MODULE", "django.slaptijack.com.settings"
)
from django.core.management import execute_from_command_line
execute_from_command_line(sys.argv)
Make sure this line is correctly pointing to django.slaptijack.com.settings
.
3. Ensure __init__.py
Files are Present
For Python to recognize a directory as a package, an __init__.py
file must be
present in that directory. Verify that your directory structure includes
__init__.py
files:
django.slaptijack.com/
django/
__init__.py
slaptijack/
__init__.py
com/
__init__.py
4. Correct the Module Name
Ensure that the module name is correctly specified. In this case, it should be:
os.environ.setdefault("DJANGO_SETTINGS_MODULE", "django.slaptijack.com.settings")
Common mistakes include:
- Typographical errors (e.g.,
slaptijack
misspelled asslaptijak
). - Incorrect directory names.
5. Verify the Python Path
Ensure that the project root directory is in the Python path. When you run
./manage.py
, Django should be able to locate the django.slaptijack.com
module. You can modify the manage.py
to include the project root in the Python
path:
import os
import sys
if __name__ == "__main__":
sys.path.insert(0, os.path.abspath(os.path.join(os.path.dirname(__file__), 'django')))
os.environ.setdefault("DJANGO_SETTINGS_MODULE", "django.slaptijack.com.settings")
from django.core.management import execute_from_command_line
execute_from_command_line(sys.argv)
6. Check for Python Version Compatibility
Ensure that you are using a compatible version of Python with Django. The error traceback indicates the use of Python 2.5, which is outdated. Consider upgrading to a more recent version of Python, as modern Django versions require Python 3.x.
Conclusion
Encountering the "No module named django.slaptijack.com" error when starting your
Django service typically indicates issues with module imports due to incorrect
paths, missing files, or misconfigurations. By following the steps outlined in
this article, you can systematically troubleshoot and resolve the issue. Ensuring
a correct project structure, accurate module naming, and proper configuration of
the DJANGO_SETTINGS_MODULE
environment variable are crucial to avoid such
import errors in Django projects.