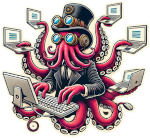
Modern distributed systems often span multiple programming languages, architectures, and runtimes. As services grow in complexity and scale, developers turn to powerful serialization frameworks and RPC (Remote Procedure Call) mechanisms that streamline inter-service communication. Two such technologies, Thrift and Protobuf, have emerged as popular solutions.
This article sets the stage for our six-part series, where we will explore how to build cross-language client/server applications using Thrift and Protobuf across three programming languages: Java, Rust, and Python. By the end of this series, you’ll understand how to define services, generate code, and implement both clients and servers that can seamlessly communicate regardless of their underlying language.
Why Thrift and Protobuf?
Thrift (originating from Facebook, now Apache Thrift) and Protobuf (from Google) both solve a similar problem: defining language-neutral data structures and enabling efficient communication across different systems. Instead of hand-rolling custom serialization logic, these frameworks leverage a schema-based approach:
- You define your data types and service interfaces using an IDL (Interface Definition Language).
- You compile these definitions into language-specific code.
- Both frameworks offer binary serialization for compact, efficient message exchange.
The difference? Thrift provides an integrated RPC system out of the box, while Protobuf focuses on data structures and often pairs with gRPC for RPC functionality. Both have robust ecosystems and support a wide range of languages, making them go-to choices for cross-language communication.
Why Java, Rust, and Python?
Choosing languages can feel arbitrary, but Java, Rust, and Python each bring unique strengths to the table:
- Java: A mature, enterprise language with a vast ecosystem and excellent tooling. Many large-scale backend systems are built in Java.
- Rust: A systems programming language that promises safety and performance. Rust is increasingly popular in high-performance, resource-constrained environments.
- Python: Known for its simplicity and readability, Python is often favored for rapid prototyping, scripting, and glue code that ties systems together.
By working through examples in all three languages, this series demonstrates the flexibility of Thrift and Protobuf. It shows how you can combine languages strategically based on the problem at hand—perhaps a high-performance Rust service talking to a Java-based data pipeline, with a Python script orchestrating it all.
What This Series Covers
Over the next several articles, we’ll move from theory to practical, hands-on examples:
- (This Article) Introduction to Thrift and Protobuf
Learn what these frameworks offer, why they matter, and how this series will use them in combination with Java, Rust, and Python. - Getting Started with Thrift (Java Server, Python Client)
We’ll define a simple Thrift service and run a Java-based server. Then, we’ll write a Python client to call the service, illustrating how to get all pieces working together. - Expanding Thrift with Rust
After setting the foundation with Thrift in Java and Python, we’ll introduce Rust. We’ll either add a Rust client calling the existing Java server or move the server-side logic into Rust. The goal is to explore interoperability and performance considerations. - Introducing Protobuf (Java Server, Python Client)
Switching gears, we’ll recreate a similar setup with Protobuf and gRPC. We’ll define.proto
files, run a gRPC server in Java, and implement a Python client. This will highlight how Protobuf (with gRPC) compares to Thrift’s integrated model. - Protobuf in Rust
We’ll integrate Rust into the Protobuf ecosystem, using tools liketonic
to generate Rust code from.proto
files. This demonstrates a similar approach to the Thrift scenario but in a Protobuf context, reinforcing how easily Rust can plug into these frameworks. - Best Practices, Advanced Topics, and Choosing the Right Tool
To wrap up, we’ll discuss schema evolution, performance optimization, testing strategies, and versioning. We’ll compare the developer experience of Thrift vs. Protobuf, and share practical insights to help you choose the right tool for your projects.
Tooling and Setup
Before you dive into later articles, consider setting up your environment:
- Thrift Compiler:
Install the Thrift compiler so you can generate code for Java, Rust, and Python.
Thrift Installation Guide - Protobuf Compiler (
protoc
):
Installprotoc
and the language-specific plugins for Java, Rust, and Python.
Protobuf Installation Guide - Language Toolchains:
- Java: Ensure you have a recent JDK and build tool (like Maven or Gradle).
- Rust: Install
rustup
andcargo
to manage Rust versions and dependencies. - Python: Set up Python 3 and
pip
for installing necessary packages.
These tools enable you to define IDLs, compile them to code, and build your client/server applications.
When to Choose Thrift or Protobuf?
While this series will guide you through using both frameworks, it’s worth considering when to choose one over the other:
- Use Thrift if:
- You want an integrated RPC system right out of the box.
- You value multiple transport and protocol options (binary, compact, JSON).
- You prefer a single tool for both serialization and RPC.
- Use Protobuf if:
- You’re already invested in the gRPC ecosystem or require advanced RPC features like bi-directional streaming.
- You appreciate a more streamlined syntax and strong versioning practices.
- You value the extensive community and tooling support around Protobuf and gRPC.
Looking Ahead
As we progress through the series, you’ll see that the concepts covered in this introduction—like IDL-based development, code generation, and RPC semantics—are not abstract. They directly inform how to architect multi-language systems that can evolve and scale. By the end of the series, you’ll be equipped with the knowledge and confidence to choose the right framework and language stack for your next distributed system project.
With this foundation in place, let’s move on to the practical side. In the next article, we’ll define a simple Thrift service, implement a Java server, and connect to it with a Python client. Stay tuned!