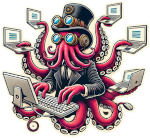
Over the course of this series, we’ve explored how to build client/server applications with Thrift and Protobuf/gRPC in three different programming languages: Java, Rust, and Python. We started with Thrift, implementing a Java server and Python client, then added Rust into the mix. Next, we recreated a similar setup with Protobuf/gRPC, again introducing Rust as a third language. In doing so, we gained firsthand experience with both frameworks’ workflows, ecosystems, and trade-offs.
In this final article, we’ll look at best practices for maintaining multi-language, multi-framework systems at scale. We’ll also cover advanced topics like schema evolution and performance considerations. Finally, we’ll provide guidance on selecting the right tool—Thrift or Protobuf/gRPC—for your particular needs.
Revisiting the Key Differences
Thrift:
- Integrated Approach: Thrift provides an all-in-one solution for serialization and RPC right in its IDL.
- Transport and Protocol Options: Built-in flexibility with multiple transports (e.g., sockets, HTTP) and protocols (binary, compact, JSON).
- Simplicity and Minimalism: The out-of-the-box experience is streamlined, though some advanced features like streaming are less prominent.
Protobuf/gRPC:
- Modular Approach: Protobuf focuses on data structures, while gRPC provides the RPC layer.
- Cloud-Native Ecosystem: gRPC integrates seamlessly with modern infrastructure—Kubernetes, service meshes, and observability tools.
- Feature-Rich RPC: Streaming, bi-directional communication, and a robust ecosystem of plugins and middleware are readily available.
Best Practices for Multi-Language RPC Systems
- Single Source of Truth for IDLs:
Whether you’re using.thrift
or.proto
files, keep them in a single repository or location accessible to all teams. This ensures everyone generates code from the same schema. - Automate Code Generation:
Incorporate code generation into your CI/CD pipeline. For instance, after any changes to.thrift
or.proto
files, run the compiler and commit generated code or distribute it through package repositories. This reduces human error and ensures consistency. - Versioning and Schema Evolution:
Over time, services evolve. Add new fields with new identifiers, rather than renumbering existing fields, to maintain backward compatibility. In Thrift and Protobuf, deprecate rather than remove fields to avoid breaking older clients. - Testing Strategies:
- Contract Tests: Ensure that both servers and clients adhere to the schema. Contract tests can catch mismatches early.
- Integration Tests: Run tests that start real servers and clients across languages to confirm end-to-end functionality.
- Load and Performance Testing: Benchmark different scenarios to ensure that adding more languages or services does not degrade performance.
- Observability and Monitoring:
- Structured Logging: Use structured logs containing request IDs, service names, and version info to debug issues effectively.
- Tracing: Implement distributed tracing (e.g., OpenTelemetry) to follow requests across services, regardless of language or framework.
- Metrics: Gather latency, throughput, and error rate metrics to spot performance bottlenecks and instability.
Advanced Topics
- Schema Evolution and Deprecation Policies:
Establish clear guidelines for how to add, remove, or change fields. Communicate changes to all teams consuming the service. Provide a grace period before removing deprecated fields. - Performance Considerations:
- Binary Formats: Both Thrift and Protobuf are efficient, but in extremely high-performance scenarios, benchmarking different protocols and frameworks may reveal subtle differences.
- Connection Management: Use connection pooling or persistent connections. gRPC supports HTTP/2 multiplexing natively, while Thrift requires choosing appropriate transports and servers.
- Streaming and Advanced RPC Features: If you need server or client streaming, gRPC offers built-in support, whereas implementing streaming in Thrift might be more manual.
- Security and Encryption:
Ensure that the chosen framework can handle secure channels (TLS/SSL). gRPC supports TLS out of the box. With Thrift, consider using HTTPs or another secure transport. - Multi-Module and Multi-Repo Setups:
Large organizations may split services into multiple repositories. Ensure consistent code generation and dependency management. For Protobuf/gRPC,buf
can help maintain consistent APIs. For Thrift, consider using a monorepo or a clear strategy for distributing IDL files.
Choosing Thrift vs. Protobuf/gRPC
When making a decision, consider:
- Integration with Existing Ecosystems:
If you’re already using gRPC for other services, adding a new service with Protobuf/gRPC might be simpler. If you have legacy systems built on Thrift, staying with Thrift reduces complexity. - Advanced RPC Features:
If you need streaming, interception, load balancing, and a full cloud-native experience, gRPC might be the better choice. If you value a simpler, integrated approach without bringing in additional frameworks, Thrift fits well. - Ecosystem Maturity and Community:
Both Thrift and Protobuf/gRPC are mature and well-supported. Consider factors like language-specific tooling, IDE support, and debugging tools. gRPC often integrates seamlessly with observability and DevOps tools popular in cloud-native environments. - Team Expertise and Velocity:
If your team is already familiar with Protobuf, it may be more cost-effective to continue using Protobuf/gRPC. If your team has significant experience with Thrift and does not require advanced gRPC features, Thrift could be more straightforward.
Looking Back and Moving Forward
Throughout this series, we saw how:
- Thrift and Protobuf/gRPC enable us to write services that talk to clients in different languages.
- With a single IDL, we can generate code for Java, Python, Rust, and more, ensuring consistency and reliability.
- Both frameworks have their own pros and cons, and your choice depends on your project’s unique requirements.
Whether you choose Thrift or Protobuf/gRPC, the foundational practices remain the same: maintain a single source of truth for schemas, automate code generation, test thoroughly, monitor your systems, and evolve your schemas with care.
Conclusion
We started with a conceptual comparison of Thrift and Protobuf, built services in Java, wrote clients in Python, integrated Rust, and explored both frameworks’ approaches to RPC. Now, you have a solid understanding of how to architect multi-language, cross-framework systems.
As you proceed with your own projects, remember that the best solutions emerge from careful evaluation of your requirements, your team’s expertise, and the capabilities of each tool. With Thrift and Protobuf/gRPC in your toolkit, you’re well-equipped to build scalable, maintainable, and high-performance distributed systems.
Thank you for following this series. We hope it provided valuable insights and empowered you to confidently design and implement cross-language, cross-framework client/server applications.