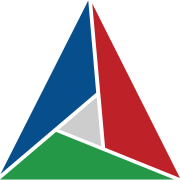
CMake is a powerful and widely used build system and project configuration tool that simplifies the process of building and managing C++ projects. With its cross-platform capabilities and extensibility, CMake has become an essential tool for C++ developers. In this technical article, we will delve deep into CMake, exploring its core concepts, best practices, and advanced features for efficiently managing C++ projects.
What Is CMake?
CMake is an open-source, cross-platform build system and project configuration tool that allows developers to define, configure, and generate build files for various build environments, such as Makefiles, Visual Studio solutions, and Xcode projects. It provides a consistent and flexible way to manage the build process, dependencies, and compiler settings.
Key Concepts
Before we dive into using CMake with C++, let's understand some essential concepts:
- CMakeLists.txt: The heart of a CMake-based project is the
CMakeLists.txt
file. It contains instructions and declarations that define the project structure, targets, and build settings. - Targets: Targets in CMake represent various entities, including executables, libraries, and custom build rules. Each target is defined with specific properties, such as source files, dependencies, and compile flags.
- Variables and Macros: CMake uses variables and macros to store and
manipulate values. Variables are defined with
set()
and accessed with${}
. Macros are similar to functions and are invoked with parentheses. - Directories: CMake organizes the project into directories, each containing
its own
CMakeLists.txt
file. Subdirectories can be added using theadd_subdirectory()
command. - Find Modules: CMake provides "Find" modules to locate external dependencies and libraries on the system. These modules simplify the integration of third-party libraries into your project.
Building a Simple C++ Project with CMake
Let's walk through the process of setting up a basic C++ project using CMake:
-
Create Project Directory Structure:
my_project/ ├── CMakeLists.txt ├── main.cpp
-
Write a CMakeLists.txt File:
cmake_minimum_required(VERSION 3.12) project(MyProject) add_executable(MyProject main.cpp)
cmake_minimum_required()
specifies the minimum required CMake version.project()
sets the project name.add_executable()
defines the executable target and its source file(s).
-
Build the Project:
mkdir build cd build cmake .. make
mkdir build
creates a build directory for out-of-source builds.cmake ..
configures the project in the build directory.make
compiles the project.
-
Run the Executable:
./MyProject
Advanced CMake Features
CMake offers several advanced features for managing C++ projects:
- External Dependencies: Use
find_package()
to locate and configure external libraries, andtarget_link_libraries()
to link them to your project. - Custom Build Rules: Define custom targets and rules with
add_custom_target()
andadd_custom_command()
. - Conditional Builds: Use
if()
andelse()
to conditionally configure build options based on variables. - Configuring Compiler Options: Set compiler flags and options with
target_compile_options()
. - Building Libraries: Create and link libraries with
add_library()
andtarget_link_libraries()
. - Generating IDE Projects: Generate project files for IDEs like Visual
Studio or Xcode with the
-G
flag when runningcmake
.
Cross-Platform Development
CMake's cross-platform capabilities make it an ideal choice for
multi-platform C++ development.
By defining platform-specific options, libraries, and settings in your
CMakeLists.txt
files, you can ensure that your code works seamlessly across
different operating systems and compilers.
Conclusion
CMake is a versatile and powerful tool for managing C++ projects, from small applications to large, complex software systems. By understanding its core concepts and exploring its advanced features, C++ developers can streamline their build processes, manage dependencies efficiently, and achieve cross-platform compatibility. Whether you're a beginner or an experienced developer, CMake is an essential tool to master in the world of C++ development.
Want to read more? Check out "Mastering CMake: A Cross-Platform Build System" by Ken Martin, Bill Hoffman, and Robert Maynard