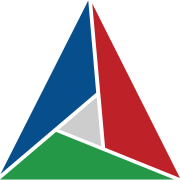
Cross-platform development is essential in the ever-diverse world of software. Whether you're targeting Windows, macOS, Linux, or even embedded systems, ensuring your C++ code runs consistently across platforms is crucial. CMake, a powerful build system and project configuration tool, is your ally in this endeavor. In this deep-dive article, we'll explore advanced techniques and best practices for achieving seamless cross-platform development with CMake and C++.
The Foundation: CMakeLists.txt
At the heart of cross-platform development with CMake is the CMakeLists.txt
file. This file defines the project structure, compilation settings, and
dependencies. To create a cross-platform project, you should:
1. Set Up Project and Version:
cmake_minimum_required(VERSION 3.12)
project(MyCrossPlatformProject)
2. Define Executable or Library Targets:
add_executable(MyApp main.cpp)
3. Platform-Independent Code:
Organize your codebase to separate platform-specific code from platform-independent code. Use conditional statements in CMake to handle platform-specific configurations:
if(UNIX)
# Unix-specific configurations
elseif(WIN32)
# Windows-specific configurations
endif()
Managing Dependencies
Handling dependencies across platforms can be complex. CMake simplifies this with
its find_package()
mechanism. For example, to include the Boost C++ Libraries:
find_package(Boost REQUIRED)
include_directories(${Boost_INCLUDE_DIRS})
target_link_libraries(MyApp ${Boost_LIBRARIES})
Remember to consider platform-specific dependencies and configure your project accordingly.
Compiler and Build Settings
CMake helps you manage compiler and build settings for different platforms:
1. Compiler Flags:
Set compiler flags using target_compile_options()
or add_compile_options()
.
For instance, to enable C++11 for all platforms:
target_compile_features(MyApp PUBLIC cxx_std_11)
2. Output Paths:
Control where build artifacts are stored using
set(CMAKE_ARCHIVE_OUTPUT_DIRECTORY ...)
and related variables.
3. Cross-Compiling:
For embedded or cross-compiling scenarios, set the CMake toolchain file using the
-DCMAKE_TOOLCHAIN_FILE
option:
cmake -DCMAKE_TOOLCHAIN_FILE=your_toolchain.cmake ..
Handling Third-Party Libraries
CMake simplifies the integration of third-party libraries, making them consistent
across platforms. Use ExternalProject
or FetchContent
for this purpose. For
example, using FetchContent
for the SQLite library:
include(FetchContent)
FetchContent_Declare(
sqlite3
GIT_REPOSITORY https://github.com/sqlite/sqlite.git
)
FetchContent_MakeAvailable(sqlite3)
Building for Different Platforms
To build for specific platforms, use the -G
option with CMake to specify the
generator:
- Windows (Visual Studio):
-G "Visual Studio 16 2019"
- macOS (Xcode):
-G Xcode
- Linux (Makefiles):
-G "Unix Makefiles"
Testing Across Platforms
CMake facilitates cross-platform testing with tools like CTest and Google Test.
To add tests to your project, use add_test()
and enable_testing()
in your
CMakeLists.txt
file.
Packaging and Distribution
Consider packaging your application for different platforms. CMake can help create platform-specific installers or packages, such as MSI installers for Windows, DMGs for macOS, or DEB/RPM packages for Linux.
Continuous Integration
To ensure code quality and cross-platform compatibility, set up a Continuous Integration (CI) system like Travis CI, Jenkins, or GitHub Actions. Automate your build and test processes for multiple platforms.
Conclusion
Cross-platform development with CMake and C++ empowers you to reach a broader audience and ensures consistent code execution across diverse environments. By mastering CMake's capabilities for managing dependencies, configuring compilers, handling third-party libraries, and testing, you'll become adept at crafting robust, cross-platform C++ applications. Whether you're developing desktop software, mobile apps, or embedded systems, CMake equips you with the tools to conquer the complexities of cross-platform development, bringing your software to users worldwide.
Want to read more? Check out "Mastering CMake: A Cross-Platform Build System" by Ken Martin, Bill Hoffman, and Robert Maynard