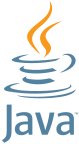
Introduction
ErrorProne is a static analysis tool for Java that
helps developers catch potential issues and improve code quality. One common
warning it provides is the
EqualsHashCode warning. This
warning suggests that the equals
method should be overridden whenever the
hashCode
method is overridden to maintain the contract between these two
methods. In this blog post, we will explore what the EqualsHashCode warning is,
why it's important, and how to resolve it effectively in your Java code.
Understanding the EqualsHashCode Warning
The EqualsHashCode warning in ErrorProne arises from the fact that in Java,
objects that are equal according to the equals
method must have the same hash
code according to the hashCode
method. Failing to maintain this contract can
lead to unexpected behavior when objects are used in collections like HashMap
or HashSet
. The warning is issued when ErrorProne detects that the hashCode
method is overridden without also overriding the equals
method in the same
class.
To illustrate the issue, consider the following code:
class Person {
private String name;
private int age;
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
In this example, the hashCode
method is overridden to generate a hash code
based on the name
and age
fields. However, the equals
method is not
overridden. This can lead to incorrect behavior when instances of the Person
class are used in data structures that rely on both methods.
Resolving the EqualsHashCode Warning
To resolve the EqualsHashCode warning and ensure that the hashCode
and equals
methods maintain their contract, follow these steps:
-
Override the
equals
Method: To address the warning, you should override theequals
method in your class. Theequals
method should compare the current object's fields with the fields of the object being compared. Here's an example:@Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; Person person = (Person) o; return age == person.age && Objects.equals(name, person.name); }
-
Implement Proper Equality Logic: In the
equals
method, ensure that you implement appropriate equality logic for your class. In the example above, we compare thename
andage
fields for equality. -
Override the
hashCode
Method: With theequals
method correctly implemented, you can now safely override thehashCode
method. ThehashCode
method should generate a hash code based on the same fields used in theequals
method. TheObjects.hash
method is a convenient way to achieve this:@Override public int hashCode() { return Objects.hash(name, age); }
-
Test Your Implementation: After making these changes, it's crucial to thoroughly test your class to ensure that the
equals
andhashCode
methods work correctly and maintain their contract.
Conclusion
The EqualsHashCode warning in ErrorProne serves as a valuable reminder to
maintain the contract between the equals
and hashCode
methods in Java. By
correctly overriding both methods and implementing proper equality logic, you can
prevent unexpected issues when using your objects in collections or when
comparing objects for equality. Resolving this warning is essential for robust
and reliable Java code.