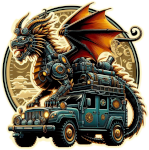
Welcome back, fellow developers! In our previous articles, we've covered the core components of LLVM, its installation, basic usage, and advanced optimizations. Now it's time to dive even deeper into the world of LLVM by exploring how to extend LLVM with custom passes and backend development. This article will guide you through the process of creating custom optimization passes and developing a custom backend for LLVM. So, open up Vim (or your preferred IDE), and let's get started!
Developing Custom Passes
Custom passes allow you to implement specific optimizations tailored to your needs. Whether you need to analyze code for specific patterns or apply unique transformations, custom passes give you the flexibility to extend LLVM's capabilities.
Writing a Custom Pass
To write a custom pass, you need to create a new C++ file and implement the pass logic. Here's a basic example of a custom pass that counts the number of instructions in each function:
#include "llvm/Pass.h"
#include "llvm/IR/Function.h"
#include "llvm/Support/raw_ostream.h"
using namespace llvm;
namespace {
struct InstructionCountPass : public FunctionPass {
static char ID;
InstructionCountPass() : FunctionPass(ID) {}
bool runOnFunction(Function &F) override {
unsigned int instructionCount = 0;
for (auto &BB : F) {
instructionCount += BB.size();
}
errs() << "Function " << F.getName() << " has " << instructionCount << " instructions.\n";
return false;
}
};
}
char InstructionCountPass::ID = 0;
static RegisterPass<InstructionCountPass> X("instr-count", "Instruction Count Pass", false, false);
Integrating Custom Passes with LLVM
To integrate your custom pass with LLVM, you need to build it as a shared library
and load it using the opt
tool. Here are the steps to do this:
-
Create a CMakeLists.txt File:
cmake_minimum_required(VERSION 3.10) project(CustomPass) set(CMAKE_CXX_STANDARD 14) find_package(LLVM REQUIRED CONFIG) include_directories(${LLVM_INCLUDE_DIRS}) add_definitions(${LLVM_DEFINITIONS}) add_library(CustomPass MODULE InstructionCountPass.cpp) target_link_libraries(CustomPass LLVM)
-
Build the Custom Pass:
mkdir build cd build cmake .. make
-
Load the Custom Pass with
opt
:opt -load ./build/CustomPass.so -instr-count input.ll -o output.ll
Example Use Case: Loop Unrolling
Loop unrolling is a common optimization that can improve performance by reducing loop overhead. Here's how you might implement a simple loop unrolling pass:
#include "llvm/Pass.h"
#include "llvm/IR/Function.h"
#include "llvm/IR/Instructions.h"
#include "llvm/Support/raw_ostream.h"
using namespace llvm;
namespace {
struct LoopUnrollPass : public FunctionPass {
static char ID;
LoopUnrollPass() : FunctionPass(ID) {}
bool runOnFunction(Function &F) override {
for (auto &BB : F) {
for (auto &I : BB) {
if (auto *Loop = dyn_cast<LoopInst>(&I)) {
// Implement loop unrolling logic here
}
}
}
return false;
}
};
}
char LoopUnrollPass::ID = 0;
static RegisterPass<LoopUnrollPass> X("loop-unroll", "Loop Unroll Pass", false, false);
Backend Development
Developing a custom backend for LLVM involves writing a target description and implementing code generation for a specific architecture. This allows you to generate machine code for custom processors or unique hardware configurations.
Overview of Backend Architecture
The LLVM backend consists of several components:
- Target Description: Defines the target architecture, including registers, instructions, and calling conventions.
- Instruction Selection: Translates LLVM IR to target-specific instructions.
- Register Allocation: Assigns physical registers to virtual registers used in the intermediate code.
- Code Emission: Generates the final machine code.
Writing a Custom Backend
Writing a custom backend is a complex task that requires in-depth knowledge of the target architecture. Here's a high-level overview of the steps involved:
-
Create Target Description Files:
- Define the target's register set, instruction set, and calling conventions.
- Implement the
TargetMachine
andTarget
classes.
-
Implement Instruction Selection:
- Use the
SelectionDAG
orGlobalISel
frameworks to translate LLVM IR to target-specific instructions. - Implement the
InstructionSelector
class.
- Use the
-
Implement Register Allocation:
- Customize the register allocation strategy to fit the target architecture.
- Implement the
RegisterAllocator
class.
-
Implement Code Emission:
- Generate the final machine code from the target-specific instructions.
- Implement the
AsmPrinter
andMCStreamer
classes.
Example: Simple Backend for a Custom Processor
Due to the complexity, providing a complete example here is impractical. However,
LLVM's documentation and the existing backends (like X86, ARM) are excellent
resources for learning how to write a custom backend. Start by examining the
lib/Target
directory in the LLVM source tree.
Testing and Validation
Testing and validation are crucial to ensure that your custom passes and backend work correctly. Here are some techniques and tools for testing LLVM components:
Unit Tests
Write unit tests for individual components of your custom pass or backend. Use LLVM's testing framework to integrate these tests.
Integration Tests
Test the end-to-end functionality by compiling and running real-world programs. Compare the results with known correct outputs to validate correctness.
Performance Benchmarks
Use performance benchmarks to measure the impact of your optimizations. Tools
like perf
and valgrind
can help analyze performance metrics and identify
bottlenecks.
Conclusion
Extending LLVM with custom passes and backend development allows you to tailor the compiler to your specific needs. In this article, we've explored how to create custom optimization passes and develop a custom backend. These techniques enable you to harness the full power of LLVM for specialized applications and unique hardware configurations.
This concludes our series on LLVM. We hope you've found these articles informative and valuable for your development projects. For more in-depth tutorials and insights into modern software development practices, stay tuned to our blog at slaptijack.com. If you have any questions or need further assistance, feel free to reach out. And remember, whether you're developing custom passes or cracking a dad joke, always strive for excellence. Happy coding!