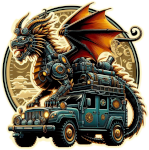
Welcome back, fellow developers! In our first article, we explored the core components of LLVM and why it's a powerful tool for modern software development. Now that you have a solid understanding of what LLVM is, it’s time to get hands-on. This article will guide you through the process of installing LLVM on various operating systems and demonstrate some basic usage scenarios to help you get started. So, fire up your preferred text editor (yes, I'm talking about Vim), and let's dive in!
Installing LLVM
On Linux
Using Package Manager
For most Linux distributions, installing LLVM and Clang is straightforward with the package manager:
sudo apt-get update
sudo apt-get install llvm clang
This method ensures you get the stable version provided by your distribution.
Building from Source
Building LLVM from source gives you the flexibility to customize the build process and ensures you are using the latest version. Here’s how you can do it:
-
Clone the LLVM project repository:
git clone https://github.com/llvm/llvm-project.git cd llvm-project
-
Create a build directory and navigate into it:
mkdir build cd build
-
Run CMake to configure the build:
cmake -G "Unix Makefiles" ../llvm
-
Compile and install LLVM:
make sudo make install
On macOS
Using Homebrew
Homebrew is a popular package manager for macOS that simplifies the installation of software. You can install LLVM using Homebrew with the following commands:
brew update
brew install llvm
To use the installed LLVM tools, you might need to add them to your PATH. You can
do this by adding the following line to your ~/.zshrc
or ~/.bash_profile
:
export PATH="/usr/local/opt/llvm/bin:$PATH"
On Windows
Using Pre-Built Binaries
LLVM provides pre-built binaries for Windows, which can be downloaded from the official LLVM website:
- Visit the LLVM releases page.
- Download the installer for the latest release.
- Run the installer and follow the instructions.
After installation, ensure that the LLVM binaries are added to your system PATH to use them from the command line.
Basic Usage of LLVM
Now that we have LLVM installed, let's explore some basic commands to get you started with this powerful toolchain.
Compiling a Simple Program with Clang
Clang is the LLVM frontend for C, C++, and Objective-C. Let's compile a simple C program to get a feel for how it works.
-
Create a file named
hello.c
with the following content:#include <stdio.h> int main() { printf("Hello, LLVM!\n"); return 0; }
-
Compile the program using Clang:
clang -o hello hello.c
-
Run the compiled program:
./hello
You should see the output:
Hello, LLVM!
Generating LLVM IR
LLVM IR (Intermediate Representation) is a powerful intermediate representation that can be used for various optimizations and transformations. To generate LLVM IR from the C program, use the following command:
clang -S -emit-llvm hello.c -o hello.ll
This command generates an LLVM IR file (hello.ll
) that contains the
intermediate representation of the program.
Viewing the LLVM IR
You can view the generated LLVM IR using any text editor (I recommend Vim, of
course). Here is an example of what the hello.ll
file might look like:
; ModuleID = 'hello.c'
source_filename = "hello.c"
target datalayout = "..."
target triple = "..."
@.str = private unnamed_addr constant [12 x i8] c"Hello, LLVM!\00", align 1
; Function Attrs: noinline nounwind optnone ssp uwtable
define i32 @main() #0 {
entry:
%call = call i32 (i8*, ...) @printf(i8* getelementptr inbounds ([12 x i8], [12 x i8]* @.str, i64 0, i64 0))
ret i32 0
}
declare i32 @printf(i8*, ...) #1
Running LLVM Optimizations
LLVM provides a suite of optimization passes that can be applied to the LLVM IR.
To run optimizations, use the opt
tool:
opt -O3 hello.ll -o hello_opt.ll
This command applies the -O3
optimization level to the LLVM IR and outputs the
optimized IR to hello_opt.ll
.
Compiling LLVM IR to Machine Code
Once you have your optimized LLVM IR, you can compile it to machine code using
the llc
tool:
llc -filetype=obj hello_opt.ll -o hello.o
This generates an object file (hello.o
) which can then be linked to create an
executable:
clang hello.o -o hello_optimized
Run the optimized executable:
./hello_optimized
You should see the same output: Hello, LLVM!
but with potentially improved
performance due to optimizations.
Conclusion
Getting started with LLVM involves setting up the toolchain on your development environment and understanding the basic commands for compiling and optimizing code. By following the steps outlined in this article, you should now have a working LLVM installation and a basic understanding of how to use it. In the next part of this series, we will delve into writing a simple compiler with LLVM, providing hands-on experience with this powerful framework.
Stay tuned to our blog at slaptijack.com for more in-depth tutorials and insights into LLVM and other modern software development practices. If you have any questions or need further assistance, feel free to reach out. And remember, whether you’re coding in Vim or VS Code, always strive to make your code as efficient and optimized as possible. Happy coding!