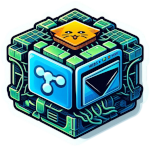
Introduction to Real-Time Tracking
Real-time location tracking has become increasingly critical in modern web applications, especially in industries such as logistics, transportation, and personal safety. This functionality allows users to track the movement of objects or individuals live, providing significant value in applications like delivery services, fleet management, and personal tracking systems.
Use Cases
- Delivery Services: Track delivery vehicles in real-time to provide accurate ETAs.
- Fleet Management: Monitor the locations and movements of vehicles within a fleet.
- Personal Tracking: Ensure the safety of individuals by tracking their real-time location.
Overview of WebSockets
What are WebSockets?
WebSockets provide a full-duplex communication channel over a single, long-lived TCP connection. Unlike traditional HTTP requests, which are unidirectional and short-lived, WebSockets allow for persistent, bidirectional communication between the client and server. This makes them ideal for real-time applications where constant data exchange is necessary.
Benefits of WebSockets for Real-Time Communication
- Low Latency: Immediate data exchange without the overhead of HTTP.
- Efficiency: Reduced bandwidth usage due to persistent connection.
- Simplicity: Simplified architecture for real-time data exchange.
GPS Data Handling
Collecting GPS Data
Modern devices equipped with GPS can provide highly accurate location data. Typically, GPS data includes latitude, longitude, altitude, and timestamps. Mobile devices, in particular, can provide this data through various APIs.
Ensuring Accuracy and Precision
- Signal Quality: Ensure the device has a clear line of sight to GPS satellites.
- Error Correction: Use Differential GPS (DGPS) or augmentation systems to improve accuracy.
- Data Smoothing: Apply algorithms to smooth out location data and reduce noise.
Implementing WebSockets for GPS Data
Setting Up a WebSocket Server
To demonstrate setting up a WebSocket server, we’ll use Node.js with the ws
library.
npm install ws
Create a WebSocket server:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', ws => {
ws.on('message', message => {
console.log(`Received message => ${message}`);
});
ws.send('Hello! Message From Server!!');
});
Client-Side Implementation
On the client side, you can create a WebSocket connection and handle GPS data.
<!DOCTYPE html>
<html>
<head>
<title>Real-Time GPS Tracking</title>
</head>
<body>
<script>
const ws = new WebSocket('ws://localhost:8080');
ws.onopen = () => {
console.log('Connected to WebSocket server');
};
ws.onmessage = (event) => {
console.log(`Received data: ${event.data}`);
};
// Simulate GPS data sending
function sendGpsData() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
const gpsData = {
latitude: position.coords.latitude,
longitude: position.coords.longitude,
timestamp: position.timestamp
};
ws.send(JSON.stringify(gpsData));
});
}
}
setInterval(sendGpsData, 1000); // Send GPS data every second
</script>
</body>
</html>
Integration with Mapping Services
Using Google Maps API
To display real-time location data on Google Maps, you need to convert GPS data into a format that Google Maps can use.
-
Load Google Maps API:
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
-
Display a Moving Marker:
<script> let map; let marker; function initMap() { map = new google.maps.Map(document.getElementById('map'), { zoom: 15, center: {lat: -34.397, lng: 150.644} }); marker = new google.maps.Marker({ position: map.getCenter(), map: map }); } function updateMarker(position) { const latLng = new google.maps.LatLng(position.latitude, position.longitude); marker.setPosition(latLng); map.setCenter(latLng); } ws.onmessage = (event) => { const gpsData = JSON.parse(event.data); updateMarker(gpsData); }; </script>
Performance and Optimization
Tips for Optimizing Real-Time Data Transmission
- Data Batching: Send multiple GPS updates in a single message to reduce overhead.
- Efficient Data Encoding: Use binary formats like Protocol Buffers for data transmission.
- Throttling Updates: Adjust the frequency of GPS updates based on application needs.
Handling Large Numbers of Connections
- Load Balancing: Distribute WebSocket connections across multiple servers.
- Scaling: Use scalable WebSocket solutions like Socket.IO with clustering or cloud-based WebSocket services.
Security Considerations
Ensuring Data Privacy and Security
- Encryption: Use TLS to encrypt WebSocket connections.
- Authentication: Implement robust authentication mechanisms to verify user identity.
- Access Control: Restrict access to WebSocket endpoints based on user roles.
Case Study
Real-World Example: Delivery Service
A delivery service application tracks the real-time location of delivery vehicles to provide accurate ETAs to customers. The application uses WebSockets to receive GPS data from delivery vehicles and displays their locations on Google Maps.
// Server-side example
const express = require('express');
const http = require('http');
const WebSocket = require('ws');
const app = express();
const server = http.createServer(app);
const wss = new WebSocket.Server({ server });
wss.on('connection', ws => {
ws.on('message', message => {
const gpsData = JSON.parse(message);
// Process GPS data (e.g., save to database, broadcast to clients)
});
});
server.listen(8080, () => {
console.log('Server is listening on port 8080');
});
Hypothetical Scenario: Personal Safety
A personal safety app uses WebSockets to track the real-time location of users and send alerts if they deviate from predefined routes. The app provides peace of mind for parents monitoring their children’s location.
Conclusion
Real-time location tracking using WebSockets and GPS data can significantly enhance the functionality and user experience of modern web applications. By understanding how to implement and optimize this technology, software engineers can build robust and scalable real-time tracking systems. For more insights and in-depth tutorials, stay tuned to our blog.
Happy coding!