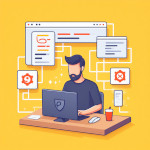
While setting up Redis as your PHP session handler unlocks tremendous speed and scalability, the journey doesn't end there. For truly robust and flexible session management, diving into its advanced features can elevate your application to new heights. This article delves into techniques that go beyond the basic setup, empowering you to leverage Redis's full potential for a secure, performant, and dynamic session management system.
1. Optimistic Locking: Concurrency Control Champion
Imagine two users editing a shared document simultaneously. Without proper conflict resolution, chaos ensues. Redis tackles this challenge with optimistic locking, preventing data race conditions in your sessions.
Here's how it works:
- During session update, a version number is associated with the session data.
- When another user attempts to update the same session, they retrieve the current version.
- If their version matches, the update proceeds. If not, it indicates another user modified the data, and their update fails, preventing conflicting changes.
This implementation can be achieved using the INCR
command to obtain the
version number and conditional SETNX
for updating only if the version matches.
Here's an example:
// Retrieve current version
$version = $redis->get('session:'.$id.':version');
// Update session data with conditional increment
$updatedData = [ /* your data modifications */ ];
$redis->setNx('session:'.$id.':data', json_encode($updatedData));
// Increment version only if update succeeded
if ($redis->incr('session:'.$id.':version') == $version + 1) {
// Update successful, proceed with further logic
} else {
// Handle conflict scenario, notify user of data being changed
}
2. Garbage Collection: Keeping Your Redis House Clean
As users log in and out, session data accumulates. To prevent your Redis database from becoming a repository of outdated sessions, implement garbage collection. This involves identifying and removing inactive sessions regularly.
Redis offers several options for garbage collection:
- Expire keys: Set expiration times for session keys so they automatically disappear after a period of inactivity.
- Lua scripting: Create custom scripts that scan for and remove expired keys based on specific criteria.
- External tools: Utilize libraries like
php-redis-gc
or dedicated Redis cluster management tools for automated garbage collection.
Choosing the right method depends on your application's needs and complexity. Remember, finding the balance between efficient purging and minimizing accidental deletion is crucial.
3. Custom Serialization: Beyond the JSON Standard
While JSON is the default serialization format for Redis, it might not be the optimal choice for every scenario. For instance, storing large arrays or binary data as JSON can bloat data size and impact performance.
Redis allows you to customize serialization by implementing custom serializers. This enables you to:
- Optimize data format: Use compact and efficient representations for specific data types.
- Enhance security: Encrypt sensitive data before serialization.
- Integrate with third-party libraries: Leverage existing serialization methods from other frameworks.
Creating a custom serializer involves implementing PHP classes that handle both serialization and deserialization of your data structures. While it requires additional development effort, the performance and security benefits can be significant.
4. Real-Time Sessions with Redis Pub/Sub
Beyond static data storage, Redis Pub/Sub unlocks real-time communication capabilities. You can leverage this for dynamic session updates and notifications:
- Session changes: Publish messages to subscribed clients whenever sessions are created, updated, or deleted.
- Live user presence: Notify other users when a specific user joins or leaves a session or online platform.
- Collaborative features: Enable real-time updates in shared documents, chats, or collaborative applications.
Implementing Pub/Sub involves subscribing clients to topic channels (e.g.,
session_updates
) and publishing messages to those channels on relevant events.
Libraries like Predis
offer convenient methods for Pub/Sub functionalities.
5. Framework Integration: A Symphony of Tools
Redis seamlessly integrates with popular PHP frameworks, further extending its capabilities:
- Laravel: Use Laravel Redis facade for easy integration with sessions, cache, and Pub/Sub features.
- Symfony: Symfony DoctrineBundle seamlessly integrates Redis as a cache backend for improved performance.
- Zend Framework: Zend Framework's Db class adapter allows using Redis for session storage and database operations.
By leveraging framework-specific integrations, you gain access to pre-built functionality and a wealth of community resources, simplifying development and maintenance.
6. Performance and Resource Optimization: Tips for a Redis Master
Optimizing resource utilization and session performance is key to a healthy Redis deployment:
- Key prefixing: Organize keys with prefixes to avoid collisions and facilitate management.
- Data partitioning: Split large session data into smaller keys to avoid exceeding Redis key size limits and optimize access patterns.
- Compression: Enable Redis compression for specific data types to reduce memory usage.
- Monitoring and logging: Track Redis metrics like memory usage, key count, and operation times to identify potential bottlenecks and optimize resource allocation.
- Clustering: Leverage Redis Cluster for horizontal scaling and improved fault tolerance when managing large user bases.
Remember, performance tuning is an ongoing process. Continuously monitor your Redis server and adapt your configuration based on observed data and changing application demands.
Conclusion
Mastering the advanced techniques offered by Redis empowers you to build a robust, secure, and performant session management system for your PHP applications. From ensuring data integrity with optimistic locking to implementing real-time updates with Pub/Sub, Redis unlocks a world of possibilities beyond the basic setup. By embracing these advanced features, integrating with available tools, and optimizing your Redis deployment, you can take your session management to the next level, ensuring a smooth, secure, and dynamic user experience for your applications.
Remember: This article provides a high-level overview of advanced Redis session techniques. Each feature warrants further exploration and adaptation based on your specific needs and application context. Don't hesitate to delve deeper into the documentation and explore community resources to unlock the full potential of Redis for your PHP session management needs.