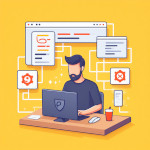
The world of web applications thrives on scalability. As your user base explodes and traffic surges, your architecture begins to groan under the pressure. One of the biggest bottlenecks in a multi-server environment? Session management. Traditional methods like file-based or cookie-based sessions struggle to keep up, leading to fragmented data, sluggish performance, and potential data loss. This is where Redis emerges as a shining beacon, offering a robust and scalable solution for session sharing and propelling your PHP application to new heights.
The Bottleneck Blues: Challenges of Multi-Server Session Management
Imagine your users seamlessly navigating through your application, unaware of the intricate dance behind the scenes. In a multi-server environment, however, session data often becomes the party pooper. Here's why:
- Data Silos: Each server holds its own set of session files or cookies, creating isolated islands of user data. This means a user's state on one server isn't shared with another, disrupting their experience and potentially forcing re-authentication.
- Performance Drain: File-based and cookie-based sessions rely on network communication and disk access, introducing significant performance overhead as user traffic scales. This translates to delayed page loads, frustration for users, and potential revenue loss.
- Scalability Limitations: As your user base grows, traditional methods buckle under the strain. Adding more servers simply adds more silos, not a unified solution for session management.
Redis to the Rescue: A Centralized Symphony for Your Sessions**
Enter Redis, the in-memory data store that revolutionizes session management. By establishing Redis as your central session store, you unlock a world of scalability, performance, and reliability:
- Shared Data Playground: Store all session data in a single Redis instance, accessible to all your servers. This breaks down data silos, ensuring a consistent user experience regardless of which server handles their request.
- Blazing Speed: Redis operates in memory, delivering lightning-fast access to session data. No more disk latency or network overheads, just instant retrieval and updates for a smooth user experience.
- Effortless Scalability: Redis clusters scale horizontally, distributing session data across multiple servers. This allows you to handle growing traffic gracefully without compromising performance or data integrity.
Different Strokes for Different Folks: Approaches to Redis Session Sharing
Redis offers two key approaches to session sharing, each with its own strengths and considerations:
- Clustering:
- Concept: Set up a Redis cluster with multiple nodes, replicating session data across the cluster for redundancy and resilience.
- Benefits: High availability and fault tolerance, ensuring session data remains accessible even if individual nodes fail.
- Considerations: Requires robust cluster management tools and additional configuration overhead.
- Replication:
- Concept: Establish a master-slave relationship between Redis servers where the master stores the data and slaves continuously replicate it.
- Benefits: Improved read performance as slaves can handle read requests, freeing up the master for writes.
- Considerations: Less fault tolerant than clustering, as data loss can occur if the master fails before replication completes.
Choosing the Right Tune: Selecting the Optimal Session Sharing Strategy
Picking the ideal approach depends on your specific needs and priorities:
- High Availability: Opt for clustering for maximum redundancy and resilience, especially for mission-critical applications.
- Performance Optimization: Consider replication if read-heavy workloads dominate your application and you can tolerate slightly less fault tolerance.
- Simplicity: If your application is smaller and ease of setup is paramount, master-slave replication might be the way to go.
Remember, choosing the right strategy is an iterative process. Monitor your application's performance and user behavior, and adapt your Redis configuration as needed.
Code Crusaders: Practical Implementation with PHP Libraries
Let's get your hands dirty! Here's a peek into using popular PHP libraries for implementing Redis session sharing:
1. Predis:
use Predis\Client;
// Configure Redis client
$redis = new Client([
'host' => '127.0.0.1',
'port' => 6379,
]);
// Session management with Predis
session_save_handler_register('Redis');
session_start();
// Access session data through $_SESSION superglobal
$_SESSION['username'] = 'johndoe';
// Destroy session when needed
session_destroy();
2. php-redis:
require_once '/path/to/vendor/autoload.php';
// Configure Redis client
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
// Session management with php-redis
$sessionHandler = new PhpRedisSessionHandler($redis);
session_set_save_handler($sessionHandler);
session_start();
// Access session data through $_SESSION superglobal
$_SESSION['username'] = 'johndoe';
// Destroy session when needed
session_destroy();
These are just basic examples, and both libraries offer extensive features for advanced session management, customization, and error handling. Refer to their respective documentation for a deeper dive.
Securing Your Shared Symphony: Keeping Your Sessions Safe
While Redis brings incredible scalability and performance, security remains paramount. Don't let your user data become a vulnerable orchestra waiting to be hijacked. Here are some essential security practices for Redis session sharing:
- Encryption: Encrypt session data at rest and in transit with libraries like Mcrypt or Sodium.
- Access Control: Implement ACLs on your Redis server to restrict access to session data based on user roles and permissions.
- Session Expiration: Set expiration times for session keys to automatically invalidate them after a period of inactivity.
- IP Binding: When possible, bind session IDs to specific user IP addresses for an extra layer of protection.
- Redis Server Security: Harden your Redis server by enforcing strict authentication and access control mechanisms.
By embracing these security best practices, you can ensure that your shared session data remains a secure fortress, safeguarding your users' trust and your application's reputation.
Beyond the Session Stage: Additional Advantages of Redis Sharing
Redis's value extends beyond session management. Its powerful data structures and capabilities can unlock additional benefits for your application:
- Cache: Leverage Redis as a central cache for frequently accessed data, significantly reducing database load and improving response times.
- Messaging: Utilize Redis Pub/Sub for real-time communication between your application components and implement features like chat or push notifications.
- Leader Election: Coordinate distributed tasks and ensure consistency across your servers with Redis's powerful leader election feature.
Exploring these additional functionalities can further optimize your application's performance and add exciting features to your user experience.
Conclusion: Scaling with Confidence - Embrace the Redis Revolution
In a world where users demand speed and seamless experiences, traditional session management methods simply don't cut it. By introducing Redis as your central session store, you unlock a new paradigm of scalability, performance, and reliability. Break down data silos, ensure consistent user experiences across servers, and handle growing traffic with ease. Remember, choosing the right session sharing strategy and applying robust security practices are key to a successful implementation. So, embrace the Redis revolution, scale your PHP application with confidence, and witness its performance soar to new heights!
This comprehensive guide has offered a deep dive into scaling your PHP application with Redis session sharing. Remember, continuous learning and experimentation are key to optimizing your Redis configuration and adapting your strategy to your evolving application needs. Don't hesitate to explore the vast resources available in the Redis community and dive deeper into specific functionalities to unlock the full potential of this powerful data store. With Redis as your ally, your PHP application is poised to conquer the challenges of scalability and deliver phenomenal experiences to your users, leaving them humming a tune of satisfaction.