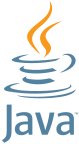
When writing Java code, it's common to call methods that return values, such as methods that read from a file, make network requests, or perform calculations. Ignoring the return value of such methods can lead to subtle bugs and unexpected behavior in your application. ErrorProne, a static analysis tool for Java, helps identify potential issues in your codebase, including the ReturnValueIgnored warning. In this blog post, we'll explore what the ReturnValueIgnored warning is, why it's essential to address it, and how to resolve it effectively.
Understanding the ReturnValueIgnored Warning
The ReturnValueIgnored warning occurs when you call a method that returns a value but fail to capture or use that return value. Consider the following example:
public class ReturnValueIgnoredExample {
public static void main(String[] args) {
String text = "Hello, World!";
text.toUpperCase(); // ReturnValueIgnored warning
}
}
In this code snippet, we have a string variable text
, and we call the
toUpperCase
method on it. However, we ignore the return value of this method,
which is a new string with all characters converted to uppercase. Ignoring this
return value means that the original text
string remains unchanged.
Why Resolve ReturnValueIgnored Warnings?
Ignoring return values may seem harmless in some cases, but it can lead to several problems:
-
Bugs: Ignoring return values can result in unexpected behavior or incorrect program logic. For instance, failing to check the return value of a file read operation may lead to data loss if the read operation fails.
-
Resource Leaks: Some methods may allocate resources that need to be explicitly released. Ignoring return values can lead to resource leaks, such as unclosed files or network connections.
-
Code Clarity: Code that ignores return values can be confusing to maintainers. It may not be clear whether the return value is intentionally ignored or if it's a mistake.
-
Security: Ignoring return values can introduce security vulnerabilities. For example, ignoring the return value of a cryptographic function can leave your application vulnerable to attacks.
Resolving ReturnValueIgnored Warnings
To resolve ReturnValueIgnored warnings effectively, consider the following strategies:
-
Assign the Return Value to a Variable: If the method's return value is important for your logic, assign it to a variable, even if you don't plan to use it immediately. This makes your intentions clear and prevents the warning:
String text = "Hello, World!"; String upperCaseText = text.toUpperCase(); // Store the result
-
Check the Return Value: If the return value indicates success or failure, check it and handle any errors appropriately. For example, when reading a file, check if the read operation was successful:
try { BufferedReader reader = new BufferedReader(new FileReader("myfile.txt")); String line = reader.readLine(); if (line != null) { // Process the line } else { // Handle end of file } reader.close(); } catch (IOException e) { // Handle the exception }
-
Use Appropriate Utility Methods: Some methods are designed for side effects and don't return meaningful values. However, they should be used judiciously. If you encounter such a method, ensure that it's the right choice for your use case.
-
Suppress the Warning (with Caution): As a last resort, you can suppress the ReturnValueIgnored warning with the
@SuppressWarnings
annotation. However, use this approach sparingly, and only when you are certain that ignoring the return value is safe:@SuppressWarnings("ReturnValueIgnored") public void someMethod() { // Your code here }
Conclusion
The ReturnValueIgnored warning in ErrorProne is a valuable tool for catching potential issues in your Java code. Ignoring return values can lead to bugs, resource leaks, and code clarity problems. By following the strategies outlined in this blog post, you can address this warning effectively and write more robust and maintainable Java code. Remember that it's essential to strike a balance between addressing the warning and maintaining code readability and simplicity.