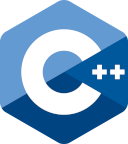
GNU Make is a powerful build automation tool widely used in software development. When combined with C++, it becomes an essential tool for compiling, linking, and managing C++ projects. This comprehensive guide will walk you through the fundamentals of using GNU Make with C++, enabling you to efficiently manage your C++ codebase and build processes.
Want to read more? Check out "Managing Projects with GNU Make" by Robert Mecklenburg
1. What is GNU Make?
Understanding Make and Makefiles
- Make is a build automation tool used to manage the compilation and linking of source code into executables or libraries.
- Makefiles are text files that contain instructions for Make, specifying how to build a project.
Why Use GNU Make with C++?
- Efficient Build Process: Make automates the build process, ensuring that only necessary files are compiled and linked, saving time.
- Dependency Management: Make tracks dependencies between source files and automatically rebuilds affected components when necessary.
- Portability: Make is cross-platform and works on various operating systems, making it suitable for multi-platform development.
- Integration: Make can be integrated into popular IDEs and text editors.
2. Setting Up Your Project
Organizing Your C++ Project Structure
- Choose a directory structure that separates source code, headers, and build artifacts.
- Organize files into logical modules or components.
Creating a Basic Makefile
- Start with a simple Makefile that includes build rules for compiling and linking a C++ program.
- Define variables for compiler options, source files, and targets.
3. Makefile Basics
Rules, Targets, and Prerequisites
- Understand the structure of a Makefile:
target: prerequisites
. - Define rules that specify how to create targets from prerequisites.
Variables in Makefiles
- Use variables to store compiler flags, source file lists, and other configuration options.
- Leverage automatic variables like
$@
,$<
, and$^
to simplify rules.
Common Makefile Commands
- Learn essential Make commands:
make
,make clean
,make all
. - Define custom targets for testing, documentation generation, and more.
4. Compiling C++ Code
Compiling Single Source Files
- Write rules to compile individual C++ source files into object files.
- Specify compiler flags and include directories.
Handling Dependencies Automatically
- Implement dependency tracking using Make's implicit rules or custom rules.
- Avoid manual dependency management to reduce errors.
Including Header Files
- Ensure header files are correctly included in your Makefile rules.
- Handle header file changes to trigger recompilation.
5. Linking Your C++ Program
Combining Object Files
- Write rules to link object files into executable or library targets.
- Specify linker flags and libraries.
Specifying Libraries and Flags
- Link external libraries using
-l
and-L
flags. - Define linker flags in Makefile variables for maintainability.
Creating Executables
- Set up rules for creating executable files from object files.
- Generate shared or static libraries when needed.
6. Advanced Makefile Techniques
Conditional Compilation
- Use conditionals in Makefiles to enable or disable specific compilation options or features.
- Customize builds for different configurations.
Recursive Make
- Handle complex projects with subdirectories by using recursive Make.
- Create Makefiles for subprojects and include them in the main Makefile.
Automatic Dependency Generation
- Implement automatic dependency generation to simplify Makefile maintenance.
- Tools like
gcc -M
or dedicated dependency generators can help.
7. Debugging and Troubleshooting
Debugging Makefiles
- Use
make
debugging options, such as-n
,-p
, and-d
, to diagnose Makefile issues. - Check for syntax errors, typos, and incorrect rules.
Common Issues and Solutions
- Address common problems like circular dependencies, missing prerequisites, and incorrect file paths.
- Document solutions and workarounds for your specific project.
Verbosity and Logging
- Implement logging and verbosity levels to aid in debugging.
- Use
echo
or custom log targets to provide feedback during the build process.
8. Optimizing Your Build Process
Parallel Builds
- Enable parallel builds with the
-j
option to accelerate compilation. - Consider system resource limitations when setting the number of jobs.
Phony Targets
- Define phony targets for non-file-related tasks like cleaning or documentation generation.
- Prevent conflicts with files of the same name.
Cleaning Up
- Create a clean target to remove build artifacts and intermediate files.
- Ensure that
make clean
effectively cleans your project.
9. Integration with IDEs and Build Systems
Using Makefiles with Visual Studio Code, CLion, and Others
- Configure your preferred IDE or text editor to recognize and work with Makefiles.
- Utilize plugins and extensions for improved integration.
Build System Integration (CMake, Autotools)
- Consider using build systems like CMake or Autotools to generate Makefiles automatically.
- These tools provide higher-level project management and cross-platform support.
10. Best Practices
Code Organization and Modularization
- Keep your codebase organized and modular to simplify build and maintenance.
- Use meaningful directory and file names.
Version Control Integration
- Integrate your project with a version control system (e.g., Git) to track changes and collaborate effectively.
- Include Makefiles and build configurations in your repository.
Documentation and Comments
- Document your Makefile structure and rules for better project understanding.
- Add comments to Makefiles to explain complex or non-obvious parts.
11. Testing and Continuous Integration
Setting Up Automated Testing
- Implement automated testing frameworks and tools (e.g., Google Test, Catch2).
- Integrate testing targets and rules into your Makefile.
Integrating with CI/CD Pipelines
- Set up Continuous Integration (CI) and Continuous Deployment (CD) pipelines to automate builds and tests.
- Use popular CI/CD platforms like Jenkins, Travis CI, or GitHub Actions.
12. Cross-Platform Development
Handling Platform-Specific Differences
- Address platform-specific code and compiler flags in your Makefiles.
- Use conditional statements to manage platform differences.
Cross-Compilation
- Configure cross-compilation in your Makefiles to build for different target architectures.
- Specify the target platform, compiler, and sysroot.
13. Resources and Further Learning
Recommended Books and Tutorials
- Explore books and online tutorials that delve deeper into GNU Make and C++ development.
Online Communities and Forums
- Join online communities and forums where you can seek help, share knowledge, and stay updated on best practices.
With this comprehensive guide, you have a solid foundation for using GNU Make with C++ to streamline your development process, manage dependencies, and create efficient build pipelines. Remember that mastering Makefiles and build automation takes practice, so don't hesitate to experiment and seek assistance from the vibrant development community.
[Disclaimer: This guide provides general information about using GNU Make with C++ and is intended for educational purposes only. While the content is based on information available as of my last update in September 2021, please consult the GNU Make documentation and other authoritative sources for the most up-to-date information. Building and maintaining complex projects may require tailored solutions and expert guidance, and readers should consult with experienced developers and experts as needed.]