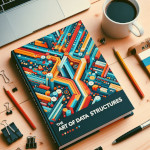
Arrays are one of the most fundamental data structures in programming, offering a simple yet powerful way to store and organize collections of elements. Whether you're a seasoned developer or just starting your programming journey, understanding arrays inside and out is crucial for building efficient and well-structured applications. This article delves into the world of arrays, demystifying concepts like indexing, multi-dimensionality, and common operations, with examples in Python, Rust, and Go.
Understanding Indexing:
An array is essentially a fixed-size container that holds elements of the same data type. Each element has a unique identifier called an index, starting from 0 in most programming languages. Accessing an element involves specifying its index within square brackets after the array name. Consider the following Python code:
numbers = [10, 20, 30, 40]
print(numbers[1]) # Output: 20 (accessing element at index 1)
Similarly, in Rust:
let numbers: [i32; 4] = [10, 20, 30, 40];
println!("{}", numbers[1]); // Output: 20
And in Go:
numbers := [4]int{10, 20, 30, 40}
fmt.Println(numbers[1]) // Output: 20
As you can see, all three languages follow the same principle of using the index to pinpoint and retrieve the desired element. Remember, attempting to access elements outside the valid index range (0 to array size-1) will result in errors.
Multi-dimensional Arrays:
Arrays offer the ability to store elements in multiple dimensions, creating grid-like structures. Imagine a table with rows and columns. Each cell in the table can be thought of as an element in a two-dimensional array. Consider this Python example:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(matrix[1][2]) # Output: 6 (accessing element at row 1, column 2)
Similar concepts apply in Rust and Go:
let matrix: [[i32; 3]; 3] = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
println!("{}", matrix[1][2]); // Output: 6
matrix := [][]int{{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}
fmt.Println(matrix[1][2]) // Output: 6
Indexing works similarly, with the first index specifying the row and the second specifying the column within that row. Multi-dimensional arrays can have more than two dimensions, creating even more complex data structures.
Common Operations:
Now that you understand indexing and multi-dimensionality, let's explore some common operations performed on arrays:
-
Traversing: Looping through each element of the array sequentially. Here's a Python example:
for number in numbers: print(number)
-
Accessing and Modifying: As seen earlier, using the index to retrieve or change the value of an element.
- Searching: Finding specific elements within the array using linear search or binary search algorithms.
- Inserting and Deleting: Adding or removing elements from the array, keeping in mind potential size constraints and shifting of elements.
- Sorting: Arranging elements in a specific order (ascending, descending) using algorithms like bubble sort, insertion sort, or merge sort.
Remember, the specific syntax and available methods for these operations might differ slightly between languages, but the underlying concepts remain similar.
In Conclusion:
Arrays provide a versatile and efficient way to organize data in your programs. Understanding indexing, multi-dimensionality, and common operations empowers you to leverage this fundamental data structure effectively. By practicing with examples in different languages like Python, Rust, and Go, you'll solidify your understanding and be well-equipped to tackle more complex data structures and algorithms in your programming journey.