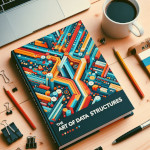
Arrays are fundamental data structures in programming, offering efficient ways to store and manage lists of elements. While the core concept remains similar across languages, their implementation and nuances vary significantly. This article delves into a comparative analysis of arrays in Python, Rust, Go, and C++, highlighting their strengths, weaknesses, and unique features.
1. Memory Allocation and Declaration:
- Python: Python arrays are dynamic, meaning their size can be adjusted
during runtime using methods like
append
andpop
. Declaration syntax utilizes square brackets[]
with initial values or without them for empty arrays. - Rust: Rust arrays are statically sized at compile time and cannot be
resized directly. They are declared using square brackets
[]
with the type and size specified. - Go: Go arrays are similar to Rust's, being statically sized and declared
using
[]
with the type and size. However, Go also providesslices
, which are dynamically sized views on underlying arrays, offering flexibility. - C++: C++ arrays are also statically sized but offer the possibility of
dynamic allocation using
new
anddelete
operators. Declarations use square brackets[]
with the type and size.
2. Data Types and Restrictions:
- Python: Python arrays are generally homogeneous, meaning all elements must
be of the same type. However, libraries like
numpy
enable multi-dimensional arrays with heterogeneous data types. - Rust: Rust enforces strict type safety, so elements in an array must share the same data type.
- Go: Go arrays have the same type restriction as Rust.
- C++: C++ allows heterogeneous arrays using unions or void pointers, but this practice is often discouraged due to potential memory safety issues.
3. Indexing and Accessing Elements:
- Python: Indexing starts from 0, and negative indices access elements from
the end. Slicing syntax
[start:end:step]
is powerful for extracting sub-arrays. - Rust: Similar to Python, indexing starts from 0 and utilizes
[]
to access elements. Rust also supports advanced indexing using ranges and iterators. - Go: Indexing is identical to Python. Slicing works slightly differently, with the "end" index being exclusive.
- C++: Indexing also starts from 0, but out-of-bounds access can lead to undefined behavior. C++ offers pointer arithmetic for more direct memory manipulation.
4. Iterating and Modifying Elements:
- Python:
for
loops or list comprehensions are commonly used for iteration. Elements can be modified directly using their index. - Rust: Iterators and methods like
iter()
oriter_mut()
are used for element access and modification. - Go: Similar to Python,
for
loops and slices work for iteration. Element modification happens directly using the index. - C++: Standard
for
loops or range-based for loops can be used. Direct modification requires careful attention to memory safety.
5. Performance and Memory Efficiency:
- Python: Dynamic nature and garbage collection can have some overhead. Homogeneous arrays offer good memory efficiency.
- Rust: Static allocation and ownership system ensure excellent performance and memory safety. Memory overhead can be higher than Python due to stricter type checking.
- Go: Similar to Rust in terms of performance and memory management. Slices provide flexibility with minimal overhead.
- C++: Potentially the most performant due to direct memory access, but requires careful coding to avoid memory leaks and security vulnerabilities.
6. Additional Features and Considerations:
- Python: Offers libraries like
numpy
for multi-dimensional arrays with advanced operations. - Rust: Has built-in methods for searching, sorting, and manipulating arrays.
- Go: Slices provide a powerful abstraction for dynamic array access.
- C++: Offers low-level control and flexibility, but requires significant expertise to use safely.
Conclusion:
The choice of language for using arrays depends on various factors like project requirements, developer familiarity, and performance needs.
- Python: Great for rapid prototyping and data analysis due to its dynamic nature and rich ecosystem of libraries.
- Rust: Ideal for memory-critical and security-sensitive applications where high performance and safety are paramount.
- Go: Well-suited for concurrent programming and building web services due to its simplicity and built-in concurrency features.
- C++: Remains a powerful choice for performance-intensive applications, but careful coding and expertise are essential to avoid potential pitfalls.
Remember, understanding the strengths and limitations of arrays in different languages allows you to make informed decisions and leverage them effectively for your specific programming needs.