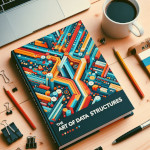
Imagine a restaurant with a single line, but instead of a chaotic first-come, first-served approach, patrons are seated based on their urgency. A pregnant woman in pain? Straight to the front. A leisurely tourist? Further back they go. This preferential treatment, applied to data, is the essence of the priority queue.
A priority queue, unlike its basic queue cousin, prioritizes elements based on assigned values. Just like in the restaurant analogy, elements with higher values (primaries) get served before those with lower ones (secondaries). This seemingly simple concept unlocks powerful applications in diverse fields, from efficient scheduling to optimizing network traffic.
Let's dive deep into the workings of this intriguing data structure, exploring its key characteristics and how it's implemented in popular programming languages like Python, Rust, and Go.
Core Principles:
- Prioritized Entries: Each element in the queue has an associated priority value (integer, date, custom score).
- Priority-Based Removal: The element with the highest priority gets removed first, regardless of its insertion order.
- Implementation Flexibility: While commonly implemented using heaps, priority queues can be built with various data structures.
- Multiple Priority Handling: Depending on the implementation, elements with the same priority might be served in specific orders (FIFO, LIFO, random).
Real-World Applications:
- Task Scheduling: Prioritize urgent tasks in operating systems or event loops.
- Graph Algorithms: Dijkstra's shortest path and Prim's minimum spanning tree rely heavily on priority queues.
- Network Traffic Management: Route data packets based on priority levels for smooth network flow.
- Huffman Coding: Compress data efficiently by prioritizing frequent symbols.
Implementation Examples:
Python:
from heapq import heappop, heappush
class PriorityQueue:
def __init__(self):
self._queue = []
def enqueue(self, item, priority):
heappush(self._queue, (priority, item))
def dequeue(self):
priority, item = heappop(self._queue)
return item
# Example usage:
pq = PriorityQueue()
pq.enqueue("Emergency", 10)
pq.enqueue("Normal", 5)
pq.enqueue("Urgent", 7)
urgent_item = pq.dequeue()
print(f"First served item: {urgent_item}") # Output: Urgent
Rust:
use std::collections::BinaryHeap;
struct Item {
priority: i32,
data: String,
}
impl PartialEq for Item {
fn eq(&self, other: &Self) -> bool {
self.priority == other.priority
}
}
impl Eq for Item {}
impl PartialOrd for Item {
fn partial_ord(&self, other: &Self) -> Option<std::cmp::Ordering> {
Some(self.priority.cmp(&other.priority))
}
}
fn main() {
let mut pq = BinaryHeap::new();
pq.push(Item { priority: 10, data: "Critical".to_string() });
pq.push(Item { priority: 5, data: "Regular".to_string() });
let next_item = pq.pop();
println!("First served item: {}", next_item.unwrap().data); // Output: Critical
}
Go:
package main
import (
"container/heap"
)
type item struct {
priority int
data string
}
type ItemQueue []item
func (pq ItemQueue) Len() int { return len(pq) }
func (pq ItemQueue) Swap(i, j int) { pq[i], pq[j] = pq[j], pq[i] }
func (pq ItemQueue) Less(i, j int) bool { return pq[i].priority > pq[j].priority }
func (pq *ItemQueue) Push(x interface{}) {
*pq = append(*pq, x.(item))
heap.Push(pq)
}
func main() {
pq := &ItemQueue{}
heap.Push(pq, item{priority: 10, data: "High"})
heap.Push(pq, item{priority: 5, data: "Low"})
first := heap.Pop(pq).(item)
println("First served item:", first.data) // Output: High
}
These examples showcase the core principle of prioritizing elements based on their assigned values. As you can see, despite the different syntax of each language, the underlying concept of the priority queue remains consistent. It's all about maintaining a dynamic order based on priorities and efficiently retrieving the element with the highest priority at any given time.
Beyond the Basics:
While implementing a basic priority queue is straightforward, there are deeper aspects to explore. These include:
- Performance Optimization: Different data structures, like Fibonacci heaps, offer faster insertion and removal times compared to traditional heaps.
- Custom Prioritization Schemes: You can tailor the priority calculation logic to suit specific needs, such as considering timestamps or dynamically changing values.
- Error Handling and Consistency: Ensuring proper handling of edge cases and maintaining data consistency during concurrent access are crucial for robust implementations.
Further Exploration:
The world of priority queues is vast and fascinating. Here are some resources to delve deeper and unlock the full potential of this versatile data structure:
- Dijkstra's Shortest Path Algorithm: https://simple.wikipedia.org/wiki/Dijkstra%27s_algorithm
- Prim's Minimum Spanning Tree Algorithm: https://en.wikipedia.org/wiki/Prim%27s_algorithm
- Huffman Coding: https://simple.wikipedia.org/wiki/Huffman_coding
- Advanced Priority Queue Implementations: https://en.wikipedia.org/wiki/Fibonacci_heap
Remember, understanding and utilizing priority queues can significantly improve the efficiency and performance of your algorithms and applications. So, go forth and conquer the queue with wisdom and priorities!