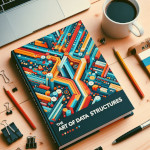
In the vast landscape of data structures, arrays stand tall as one of the most fundamental and widely used tools. Their simplicity belies their immense power, allowing them to efficiently store and manipulate collections of elements. Whether you're building dynamic websites or crunching scientific data, understanding arrays is crucial for any programmer.
What is an Array?
Imagine a bookshelf neatly holding a row of books. An array is similar, but instead of books, it stores elements of the same data type, like numbers, characters, or even other data structures. Each element has a unique address, called an index, starting from 0 in most languages. This index acts as a key, allowing you to access and manipulate individual elements with ease.
Creating and Accessing Elements:
Let's dive into code examples to grasp the essence of arrays. Here's how to create and access elements in Python, Rust, and Go:
Python:
# Create an array of numbers
numbers = [1, 2, 3, 4, 5]
# Access the second element (index 1)
second_element = numbers[1]
# Print the accessed element
print(second_element) # Output: 2
Rust:
// Create an array of characters
letters: [char; 5] = ['a', 'b', 'c', 'd', 'e'];
// Access the third element (index 2)
third_letter = letters[2];
// Print the accessed element
println!("{}", third_letter); // Output: c
Go:
// Create an array of integers
numbers := [5]int{1, 2, 3, 4, 5}
// Access the fourth element (index 3)
fourth_number := numbers[3]
// Print the accessed element
fmt.Println(fourth_number) // Output: 4
As you can see, the syntax might differ slightly, but the concept remains the same: use the index within square brackets to pinpoint and retrieve elements.
Beyond the Basics:
Arrays offer more than just simple storage. You can:
- Loop through all elements: Iterate over each element sequentially using loops, processing them individually.
- Modify elements: Change the value stored at a specific index.
- Search for elements: Find specific elements within the array using linear or binary search algorithms.
- Create multi-dimensional arrays: Imagine multiple bookshelves stacked together; these represent arrays with two or more dimensions, useful for storing complex data like grids or images.
Strengths and Limitations:
The key advantages of arrays lie in their efficiency for random access (constant time complexity) and simplicity of implementation. However, they also have limitations:
- Fixed size: Once created, the size of an array cannot be changed dynamically.
- Insertion and deletion: Inserting or deleting elements in the middle can be expensive, often requiring shifting other elements.
When to Use Arrays:
Arrays shine when you need to:
- Store a fixed collection of elements of the same data type.
- Access elements efficiently using their index.
- Perform simple operations like searching or sorting.
Conclusion:
Arrays might seem like a basic building block, but their versatility and efficiency make them an essential tool for any programmer. By understanding their concepts and limitations, you can harness their power in diverse scenarios, laying a solid foundation for your programming journey.