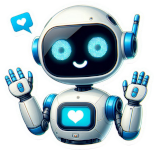
Creating a chatbot has become an essential skill for modern software engineers, especially with the rise of AI-driven applications. In this series, we'll dive deep into building a chatbot using Python and the OpenAI API. Below is an outline of the articles we'll cover:
Article 1: Introduction to Chatbots and the OpenAI API
In the first article, we'll explore the fundamentals of chatbots and how they have evolved over time. We'll introduce the OpenAI API, discuss its capabilities, and set the stage for what we'll build throughout the series.
Key Topics
- The history and evolution of chatbots
- Understanding different types of chatbots
- Overview of the OpenAI API and its features
- Setting up expectations for the series
Article 2: Setting Up Your Development Environment
Before we start coding, we'll need to set up our development environment. I'll walk you through installing Python, configuring VS Code (my preferred IDE), and setting up virtual environments.
Key Topics
- Installing Python 3.x
- Configuring VS Code for Python development
- Setting up a virtual environment with
venv
- Installing necessary Python packages
Article 3: Making Your First API Call with OpenAI
In this article, we'll make our first API call to OpenAI. We'll register for an API key, learn how to authenticate requests, and understand the basics of sending and receiving data.
Key Topics
- Registering and obtaining an OpenAI API key
- Understanding API authentication and security practices
- Writing a simple Python script to interact with the API
- Parsing and handling API responses
Article 4: Building a Basic Chatbot Interface
Now that we can communicate with the OpenAI API, we'll build a basic command-line interface for our chatbot. This will allow users to input messages and receive responses.
Key Topics
- Designing a simple user interface in the terminal
- Handling user input and output in Python
- Integrating the interface with OpenAI API calls
- Testing the chatbot's conversational abilities
Article 5: Enhancing the Chatbot with Contextual Awareness
A good chatbot maintains context. In this article, we'll implement features that allow our chatbot to remember previous interactions, making conversations more coherent.
Key Topics:
- Understanding the importance of context in conversations
- Storing and managing conversation history
- Modifying API calls to include contextual information
- Improving response relevance and coherence
Article 6: Customizing the Chatbot's Personality
We'll explore how to adjust the chatbot's tone and style to make interactions more engaging. Customizing the chatbot's personality can make it more suitable for specific applications.
Key Topics
- Using OpenAI's parameters (
temperature
,max_tokens
, etc.) - Crafting custom prompts for desired behaviors
- Implementing user profiles for personalized experiences
- Ethical considerations in chatbot personality design
Article 7: Deploying Your Chatbot as a Web Application
In the final article, we'll deploy our chatbot as a web application using Flask. We'll discuss hosting options and best practices for deployment.
Key Topics:
- Introduction to Flask for web development
- Building a web interface for the chatbot
- Securing API keys and handling secrets
- Deployment options (Heroku, AWS, Docker)
Conclusion: Next Steps and Additional Resources
We'll wrap up the series by summarizing what we've learned and suggesting next steps for further development. Additional resources will be provided for continued learning.
By the end of this series, you'll have a fully functional chatbot built with Python and the OpenAI API. Whether you're enhancing your skill set or laying the groundwork for more complex AI projects, this series will equip you with the knowledge and tools you need.
For more tutorials and insights on boosting your developer productivity, be sure to check out slaptijack.com.