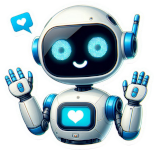
You've built a sophisticated chatbot using Python and the OpenAI API—congratulations! Now, it's time to share your creation with the world. In this article, we'll explore how to deploy your chatbot as a web application using Flask, a lightweight web framework for Python. We'll discuss setting up the Flask app, integrating your chatbot, and deploying it to a hosting service like Heroku or AWS. By the end of this tutorial, your chatbot will be accessible to users through a web interface.
Why Deploy Your Chatbot as a Web Application?
Accessibility
Deploying your chatbot as a web application makes it accessible to anyone with an internet connection. Users won't need to install anything; they can interact with your chatbot directly from their browsers.
User Experience
A web interface provides a more user-friendly experience compared to a command-line application. You can design the interface to be intuitive and engaging.
Scalability
Web applications can be scaled to handle multiple users simultaneously, especially when deployed on cloud platforms.
Introduction to Flask
What Is Flask?
Flask is a micro web framework written in Python. It's known for its simplicity and flexibility, making it ideal for small to medium-sized projects.
Key Features
- Lightweight: Minimal setup required.
- Extensible: Easily integrates with extensions for added functionality.
- Pythonic: Designed to make it easy to write Python code.
Setting Up Your Flask Application
Step 1: Install Flask
First, ensure your virtual environment is activated. Then, install Flask:
pip install flask
Step 2: Create a New Python Script
Create a new file called app.py
in your project directory.
touch app.py
Step 3: Basic Flask App Structure
Add the following code to app.py
:
from flask import Flask, render_template, request
import os
import openai
from dotenv import load_dotenv
app = Flask(__name__)
load_dotenv()
openai.api_key = os.getenv('OPENAI_API_KEY')
Step 4: Create a Simple Route
Define a route for the home page:
@app.route('/')
def index():
return render_template('index.html')
Integrating the Chatbot with Flask
Step 1: Modify the generate_response
Function
Adapt the generate_response
function to work with Flask sessions:
def generate_response(prompt, context):
context.append({"role": "user", "content": prompt})
try:
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=context,
temperature=0.7,
)
answer = response.choices[0].message['content'].strip()
context.append({"role": "assistant", "content": answer})
return answer, context
except openai.error.OpenAIError as e:
print(f"An error occurred: {e}")
return "I'm sorry, but I couldn't process that.", context
Step 2: Handle User Input
Create a route to handle form submissions:
@app.route('/chat', methods=['POST'])
def chat():
user_input = request.form['user_input']
context = request.cookies.get('context')
if context:
context = json.loads(context)
else:
context = [{"role": "system", "content": "You are a helpful assistant."}]
response, context = generate_response(user_input, context)
resp = make_response(render_template('index.html', response=response))
resp.set_cookie('context', json.dumps(context))
return resp
Step 3: Import Necessary Modules
Add the following imports at the top of app.py
:
import json
from flask import make_response
Creating the HTML Template
Step 1: Set Up the Templates Directory
Create a templates
folder in your project directory:
mkdir templates
Step 2: Create index.html
Create an index.html
file inside the templates
folder:
touch templates/index.html
Step 3: Basic HTML Structure
Add the following HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Chatbot Web Interface</title>
</head>
<body>
<h1>Chat with the Chatbot</h1>
<form action="/chat" method="post">
<input type="text" name="user_input" placeholder="Type your message here">
<input type="submit" value="Send">
</form>
{% if response %}
<p><strong>Chatbot:</strong> {{ response }}</p>
{% endif %}
</body>
</html>
Step 4: Enhancing the Interface with CSS
You can add CSS to make the interface more appealing. Create a static
folder
and add a styles.css
file.
mkdir static
touch static/styles.css
Link the CSS in your index.html
:
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='styles.css') }}">
Running the Flask App Locally
Step 1: Set the Flask App Environment Variable
On Windows:
set FLASK_APP=app.py
On macOS/Linux:
export FLASK_APP=app.py
Step 2: Run the App
flask run
Step 3: Access the App
Open your web browser and navigate to http://127.0.0.1:5000/
.
Handling Sessions and Context
Using Flask Sessions
We'll use Flask's session management to store the conversation context.
Step 1: Configure Secret Key
Add a secret key to your Flask app:
app.secret_key = os.getenv('SECRET_KEY', 'your_secret_key')
Step 2: Modify Routes to Use Sessions
Update the chat
route:
from flask import session
@app.route('/chat', methods=['POST'])
def chat():
user_input = request.form['user_input']
if 'context' not in session:
session['context'] = [{"role": "system", "content": "You are a helpful assistant."}]
context = session['context']
response, context = generate_response(user_input, context)
session['context'] = context
return render_template('index.html', response=response)
Deploying to Heroku
Step 1: Install Gunicorn
Gunicorn is a Python WSGI HTTP server for UNIX.
pip install gunicorn
Step 2: Create a Procfile
In your project root directory, create a Procfile
:
touch Procfile
Add the following line:
web: gunicorn app:app
Step 3: Create requirements.txt
Generate a requirements.txt
file:
pip freeze > requirements.txt
Step 4: Sign Up for Heroku
If you don't have an account, sign up at Heroku.
Step 5: Install the Heroku CLI
Download and install the Heroku CLI.
Step 6: Login to Heroku
heroku login
Step 7: Create a New Heroku App
heroku create your-app-name
Step 8: Deploy to Heroku
Initialize a git repository and commit your code:
git init
git add .
git commit -m "Initial commit"
Set the Heroku remote and push:
heroku git:remote -a your-app-name
git push heroku master
Step 9: Set Environment Variables
Set your OpenAI API key and Flask secret key:
heroku config:set OPENAI_API_KEY='your-openai-api-key'
heroku config:set SECRET_KEY='your-secret-key'
Step 10: Open Your App
heroku open
Deploying to AWS Elastic Beanstalk
Alternatively, you can deploy your app to AWS Elastic Beanstalk.
Step 1: Install the AWS CLI
Install the AWS CLI.
Step 2: Configure AWS Credentials
aws configure
Step 3: Initialize Elastic Beanstalk
eb init -p python-3.7 your-app-name
Step 4: Create an Environment
eb create your-env-name
Step 5: Deploy
eb deploy
Step 6: Set Environment Variables
In the AWS Console, navigate to Elastic Beanstalk, select your environment, and
add environment variables for OPENAI_API_KEY
and SECRET_KEY
.
Securing API Keys and Handling Secrets
Best Practices
- Use Environment Variables: Never hardcode sensitive information.
- Version Control: Exclude files like
.env
from version control by adding them to.gitignore
. - Config Vars: Use the hosting platform's configuration settings to manage environment variables.
Optimizing for Production
Logging
Implement logging to monitor your application's performance.
import logging
logging.basicConfig(level=logging.INFO)
Error Handling
Ensure that your app gracefully handles errors.
@app.errorhandler(500)
def internal_error(error):
return "An unexpected error occurred.", 500
Scalability
Consider using a database or caching system if you expect high traffic.
Recommended Tools and Accessories
Domain Name Registration
Give your chatbot a custom domain name. Use Namecheap for affordable domain registration.
SSL Certificates
Secure your web application with SSL certificates. Services like Let's Encrypt offer free SSL certificates.
Monitoring Tools
- Sentry: For real-time error tracking.
- New Relic: For application performance monitoring.
Books on Web Development
"Flask Web Development: Developing Web Applications with Python" by Miguel Grinberg.
Conclusion
Deploying your chatbot as a web application is a significant milestone. You've transformed a command-line program into a user-friendly web app accessible to anyone. We've covered setting up a Flask application, integrating your chatbot, and deploying it to Heroku or AWS. With your chatbot now live, you can start gathering user feedback and continue refining its features.
This deployment not only makes your chatbot more accessible but also opens doors for further enhancements like adding user authentication, integrating databases, or even creating a mobile app interface.
For more tutorials and insights on boosting your developer productivity, be sure to check out slaptijack.com.