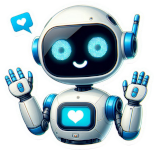
Creating a chatbot that can interact naturally with users is an exciting challenge. In our previous articles, we've built a functional chatbot using Python and the OpenAI API. Now, it's time to take it a step further by customizing the chatbot's personality. By adjusting its tone, style, and behavior, we can make interactions more engaging and tailor the chatbot to specific applications. In this article, we'll explore techniques to customize your chatbot's personality, implement different personas, and discuss the ethical considerations involved.
Why Customize the Chatbot's Personality?
Enhancing User Engagement
A chatbot with a well-defined personality can make conversations more enjoyable and engaging. It helps in building rapport with users and keeps them coming back.
Aligning with Brand Identity
For businesses, aligning the chatbot's personality with the brand's identity can enhance the overall user experience and reinforce brand values.
Improving User Satisfaction
A chatbot that communicates in a style that resonates with the target audience can improve user satisfaction and effectiveness.
Understanding the Role of the System Message
When using OpenAI's Chat Completion API, the system message plays a crucial role in setting the behavior and personality of the chatbot.
What Is the System Message?
The system message helps set the behavior of the assistant. It can be used to instruct the chatbot to adopt a specific tone, style, or role.
Example:
context = [
{"role": "system", "content": "You are a helpful and friendly assistant."}
]
By modifying the content of the system message, we can influence how the chatbot responds.
Techniques for Customizing Personality
Defining the Chatbot's Role
Specify the chatbot's role clearly in the system message.
-
Friendly Assistant:
{"role": "system", "content": "You are a friendly and cheerful assistant who loves to help people."}
-
Professional Advisor:
{"role": "system", "content": "You are a professional advisor who provides concise and accurate information."}
-
Humorous Companion:
{"role": "system", "content": "You are a witty companion who tells jokes and makes conversations fun."}
Adjusting Tone and Language
Use descriptive language in the system message to adjust the chatbot's tone.
-
Formal Tone:
{"role": "system", "content": "You are a formal and polite assistant who uses professional language."}
-
Casual Tone:
{"role": "system", "content": "You are a casual assistant who speaks in a relaxed and friendly manner."}
Setting Behavioral Guidelines
Provide specific instructions on how the chatbot should behave.
-
Avoiding Certain Topics:
{"role": "system", "content": "You are an assistant who avoids discussing politics or personal opinions."}
-
Encouraging Certain Behaviors:
{"role": "system", "content": "You are an assistant who always provides detailed explanations and examples."}
Incorporating Domain Knowledge
If your chatbot is meant for a specific field, you can instruct it accordingly.
-
Tech Support Specialist:
{"role": "system", "content": "You are a tech support specialist with expertise in networking and system administration."}
-
Fitness Coach:
{"role": "system", "content": "You are a fitness coach who provides workout tips and nutritional advice."}
Implementing Different Personalities
Let's implement a feature that allows switching between different personalities.
Step 1: Defining Personalities
Create a dictionary to store different system messages.
personalities = {
"friendly": "You are a friendly and cheerful assistant who loves to help people.",
"professional": "You are a professional advisor who provides concise and accurate information.",
"humorous": "You are a witty companion who tells jokes and makes conversations fun.",
"geeky": "You are a tech enthusiast who loves Vim and is opinionated about its superiority over Emacs."
}
Step 2: Modifying the Chat Function
Allow the user to select a personality at the start or change it during the conversation.
def chat():
print("Welcome to the Chatbot! Type 'help' for a list of commands.")
selected_personality = "friendly" # Default personality
context = [{"role": "system", "content": personalities[selected_personality]}]
while True:
user_input = input("You: ")
if user_input.lower() in ['exit', 'quit']:
print("Chatbot: Goodbye!")
break
if user_input.lower() == 'help':
print("Available commands:\n - exit: Quit the chatbot\n - help: Show this help message\n - change personality: Change the chatbot's personality\n - reset: Reset the conversation context")
continue
if user_input.lower() == 'change personality':
print("Available personalities:", ", ".join(personalities.keys()))
selected_personality = input("Enter the personality you want: ").strip()
if selected_personality in personalities:
context = [{"role": "system", "content": personalities[selected_personality]}]
print(f"Chatbot: Personality changed to {selected_personality}.")
else:
print("Chatbot: Invalid personality selected.")
continue
if user_input.lower() == 'reset':
context = [{"role": "system", "content": personalities[selected_personality]}]
print("Chatbot: The conversation has been reset.")
continue
if not user_input.strip():
print("Chatbot: Please say something so I can assist you.")
continue
response, context = generate_response(user_input, context)
print(f"Chatbot: {response}")
Step 3: Testing Different Personalities
Run the chatbot and try switching between personalities.
Sample Interaction:
You: change personality
Available personalities: friendly, professional, humorous, geeky
Enter the personality you want: geeky
Chatbot: Personality changed to geeky.
You: What's your favorite text editor?
Chatbot: Without a doubt, Vim is the superior text editor. Its efficiency and modal editing make it unbeatable compared to Emacs.
You: Why do you prefer Vim over Emacs?
Chatbot: Vim's simplicity and speed allow for a more seamless coding experience. Plus, who needs a kitchen sink when you can have a razor-sharp Swiss Army knife?
Using Prompts to Guide Behavior
Sometimes, adjusting the system message isn't enough. You can also guide the chatbot's behavior by crafting prompts within the conversation.
Example: Encouraging Detailed Responses
user_input = "Explain how neural networks work."
context.append({"role": "user", "content": user_input + " Provide a detailed explanation with examples."})
Example: Limiting Responses
user_input = "Tell me about quantum computing."
context.append({"role": "user", "content": user_input + " Explain it in simple terms suitable for a beginner."})
Ethical Considerations in Personality Customization
Avoiding Harmful Behavior
Ensure that the chatbot does not produce offensive, discriminatory, or harmful content.
- Content Filtering: Implement content filters to prevent inappropriate responses.
- Policy Compliance: Follow OpenAI's usage policies to ensure ethical use.
Transparency
Inform users about the chatbot's nature and any limitations it may have.
- Disclosure: Let users know they are interacting with an AI.
- Limitations: Be upfront about the chatbot's capabilities and knowledge cutoff dates.
Privacy
Respect user privacy by not storing or misusing personal information.
Testing and Refining the Chatbot's Personality
Collecting Feedback
- User Testing: Have users interact with the chatbot and provide feedback.
- Surveys: Use surveys to gather opinions on the chatbot's personality and helpfulness.
Iterative Refinement
- Adjust System Messages: Modify the system message based on feedback.
- Fine-Tuning: Consider fine-tuning the model with custom data if needed.
Recommended Tools and Accessories
To further enhance your chatbot development experience:
Text Editors and IDEs
- Vim: For those who appreciate efficiency, Vim is unparalleled. If you're new to Vim, check out the book "Practical Vim: Edit Text at the Speed of Thought" by Drew Neil.
- VS Code Vim Extension: If you prefer VS Code but love Vim's keybindings, the VSCodeVim extension brings the best of both worlds.
Books on Conversational AI
- "Designing Bots: Creating Conversational Experiences" by Amir Shevat.
AI Ethics Resources
- "AI Ethics" by Mark Coeckelbergh offers insights into the ethical considerations of AI development.
Conclusion
Customizing your chatbot's personality can significantly enhance user engagement and satisfaction. By carefully crafting the system messages and prompts, you can tailor the chatbot to suit different applications and audiences. Remember to consider the ethical implications of personality customization, ensuring that your chatbot is both helpful and respectful.
In the next article, we'll explore deploying your chatbot as a web application using Flask. We'll discuss hosting options and best practices for deployment, bringing your chatbot to a broader audience.
For more tutorials and insights on boosting your developer productivity, be sure to check out slaptijack.com.