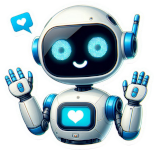
The rise of artificial intelligence has revolutionized the way we interact with technology. One of the most fascinating developments in this arena is the chatbot - a program designed to simulate conversation with human users. If you've ever chatted with customer support online or asked Siri a question, you've interacted with a chatbot. In this article, we'll delve into the world of chatbots, explore their evolution, and introduce you to the powerful OpenAI API that makes building sophisticated chatbots more accessible than ever.
What Are Chatbots?
At their core, chatbots are software applications that mimic written or spoken human speech for the purpose of simulating a conversation. They can be as simple as rule-based programs that respond to specific keywords or as complex as AI-driven systems that understand context and generate human-like responses.
The Evolution of Chatbots
- Rule-Based Chatbots: Early chatbots operated on predefined scripts. They could respond to specific inputs but lacked the ability to understand context or handle unexpected queries.
- AI-Powered Chatbots: With advancements in machine learning and natural language processing (NLP), modern chatbots can understand context, learn from interactions, and provide more accurate and helpful responses.
Why Chatbots Matter
- 24/7 Availability: Chatbots can provide support and information around the clock.
- Scalability: They can handle multiple conversations simultaneously, making them invaluable for businesses.
- Cost-Effective: Automating customer interactions reduces the need for large support teams.
Introducing the OpenAI API
The OpenAI API is a cloud-based platform that provides access to advanced AI models developed by OpenAI. These models can understand and generate natural language, making them ideal for building chatbots.
Key Features
- Natural Language Understanding: The API can comprehend and generate human-like text based on the input it receives.
- Versatility: Suitable for a wide range of applications, from drafting emails to writing code.
- Ease of Use: Designed to be developer-friendly with comprehensive documentation and support.
How It Works
At a high level, you send a prompt to the API, and it returns a generated response. The sophistication of the response depends on the model used and the parameters set.
import openai
openai.api_key = 'YOUR_API_KEY'
response = openai.Completion.create(
engine="text-davinci-003",
prompt="Hello, how are you?",
max_tokens=5
)
print(response.choices[0].text.strip())
This simple example sends a greeting to the API and prints the response.
Setting the Stage for Our Chatbot
Over the next series of articles, we'll guide you through building your own chatbot using Python and the OpenAI API. We'll start from the basics and gradually move towards creating a sophisticated, context-aware chatbot.
What You'll Learn
- Setting Up Your Development Environment: Installing Python, configuring your IDE, and managing dependencies.
- Interacting with the OpenAI API: Making API calls, handling responses, and managing authentication.
- Building the Chatbot Interface: Creating a user-friendly interface for interactions.
- Adding Contextual Awareness: Implementing features that allow the chatbot to remember previous interactions.
- Customizing Personality: Adjusting the chatbot's tone and style to suit your needs.
- Deployment: Turning your chatbot into a web application and deploying it for others to use.
Tools and Technologies
- Python: Our programming language of choice due to its simplicity and extensive libraries.
- OpenAI API: The backbone of our chatbot's conversational capabilities.
- VS Code: My preferred Integrated Development Environment (IDE) for writing and debugging code.
Preparing for Development
Before we dive into coding, let's make sure you have everything you need.
Python Installation
Ensure you have Python 3.x installed on your machine. You can download it from the official website.
Setting Up VS Code
Download and install Visual Studio Code. It's a versatile editor that supports a wide range of extensions, including those for Python development.
Virtual Environments
Using virtual environments helps manage dependencies and keep your projects organized.
# Create a virtual environment
python -m venv chatbot_env
# Activate the virtual environment
# On Windows
chatbot_env\Scripts\activate
# On macOS/Linux
source chatbot_env/bin/activate
Required Python Packages
We'll be using the openai
package to interact with the API.
pip install openai
Understanding the OpenAI API in Depth
To make the most of the API, it's essential to understand its components.
API Endpoints
- Completion Endpoint: Generates text based on a given prompt.
- Chat Endpoint: Designed specifically for chatbot applications, allowing for more interactive conversations.
Authentication and Security
Always keep your API keys secure. Avoid hardcoding them into your scripts or sharing them publicly. Use environment variables or configuration files that are excluded from version control.
import os
import openai
openai.api_key = os.getenv('OPENAI_API_KEY')
Pricing Considerations
The OpenAI API uses a pay-as-you-go model. Be mindful of the tokens used during development to manage costs effectively.
Ethical Considerations
As we build AI-driven applications, it's crucial to consider the ethical implications.
- Data Privacy: Ensure that user data is handled securely and ethically.
- Bias and Fairness: Be aware of potential biases in AI responses and take steps to mitigate them.
- Transparency: Inform users when they are interacting with a chatbot.
Conclusion
Building a chatbot is an exciting journey that combines programming skills with cutting-edge AI technology. With the OpenAI API and Python, you have all the tools you need to create a chatbot that can understand and generate human-like text.
In the next article, we'll set up our development environment and prepare everything we need to start coding. Stay tuned as we embark on this fascinating project together.
For more tutorials and insights on boosting your developer productivity, be sure to check out slaptijack.com.