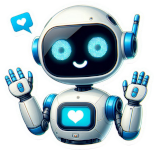
Before diving into building our chatbot using Python and the OpenAI API, it's crucial to set up a development environment that is both efficient and comfortable to work with. A well-configured environment can significantly boost your productivity and make the coding process smoother. In this article, we'll walk through installing Python, configuring Visual Studio Code (VS Code), setting up a virtual environment, and installing the necessary Python packages.
Installing Python 3.x
The first step is to ensure that Python 3.x is installed on your system.
For Windows Users
- Download the Installer: Visit the official Python website and download the latest Python 3.x installer.
- Run the Installer: Execute the downloaded file. Make sure to check the box that says "Add Python 3.x to PATH".
-
Verify Installation: Open the Command Prompt and run:
python --version
You should see the installed Python version.
For macOS Users
-
Use Homebrew: If you have Homebrew installed, you can install Python by running:
brew install python
-
Verify Installation:
python3 --version
For Linux Users
-
Using Package Manager:
sudo apt-get update sudo apt-get install python3 python3-pip
-
Verify Installation:
python3 --version
Setting Up Visual Studio Code (VS Code)
VS Code is my preferred Integrated Development Environment (IDE) due to its versatility and extensive range of extensions.
Installation
- Download VS Code: Visit the official website and download the installer suitable for your operating system.
- Run the Installer: Follow the on-screen instructions to complete the installation.
Configuring VS Code for Python Development
-
Install the Python Extension:
- Open VS Code.
- Go to the Extensions view by clicking on the square icon on the sidebar or
pressing
Ctrl+Shift+X
. - Search for "Python" and install the extension by Microsoft.
-
Configure Python Interpreter:
- Press
Ctrl+Shift+P
to open the Command Palette. - Type "Python: Select Interpreter" and select the Python 3.x interpreter.
- Press
-
Enable Linting and Formatting:
-
Install linting tools like
pylint
:pip install pylint
-
Configure settings in
settings.json
:"python.linting.enabled": true, "python.linting.pylintEnabled": true, "editor.formatOnSave": true
-
Setting Up a Virtual Environment
Using a virtual environment isolates your project’s dependencies and ensures that packages installed for one project won't affect others.
Creating a Virtual Environment
Navigate to your project directory and run:
# Create a directory for your project
mkdir chatbot_project
cd chatbot_project
# Create a virtual environment named 'venv'
python -m venv venv
Activating the Virtual Environment
-
On Windows:
venv\Scripts\activate
-
On macOS/Linux:
source venv/bin/activate
You should now see (venv)
preceding your command prompt, indicating that the
virtual environment is active.
Deactivating the Virtual Environment
When you're done working, you can deactivate the environment by running:
deactivate
Installing Necessary Python Packages
With the virtual environment activated, install the packages we'll need.
Installing the OpenAI Package
The openai
package allows us to interact with the OpenAI API seamlessly.
pip install openai
Installing Other Useful Packages
While not mandatory, the following packages can enhance your development experience:
-
Requests: For making HTTP requests.
pip install requests
-
Python Dotenv: For managing environment variables.
pip install python-dotenv
Managing Environment Variables
Storing sensitive information like API keys in your code is a bad practice. Instead, use environment variables.
Setting Up .env
File
- Create a
.env
File in your project root directory. -
Add Your OpenAI API Key:
OPENAI_API_KEY='your-api-key-here'
Loading Environment Variables in Python
Use python-dotenv
to load variables from the .env
file.
from dotenv import load_dotenv
import os
load_dotenv()
openai_api_key = os.getenv('OPENAI_API_KEY')
Configuring Git for Version Control
Version control is essential for any project.
Initializing a Git Repository
git init
Creating a .gitignore
File
Exclude unnecessary files and directories from your repository.
venv/
.env
__pycache__/
Customizing VS Code Settings for the Project
You can create workspace-specific settings in .vscode/settings.json
.
Example Settings
{
"python.pythonPath": "venv/bin/python",
"python.linting.pylintEnabled": true,
"python.linting.enabled": true,
"python.envFile": "${workspaceFolder}/.env"
}
Extensions to Enhance Productivity
Recommended VS Code Extensions
- GitLens: Enhances Git capabilities within VS Code.
- Prettier: An opinionated code formatter.
- Bracket Pair Colorizer: Helps in identifying matching brackets.
Recommended Tools and Accessories
To optimize your coding experience, consider investing in some hardware upgrades.
Mechanical Keyboard
A responsive keyboard can make coding more enjoyable. The Das Keyboard Model S Professional is a solid choice for developers.
Ergonomic Chair
Long coding sessions require comfort. The Herman Miller Aeron Ergonomic Chair provides excellent support.
Dual Monitor Setup
Having more screen real estate can boost productivity. Pairing two ASUS ProArt Displays can give you ample space for coding and documentation.
Testing Your Setup
Let's write a simple script to ensure everything is working correctly.
Test Script: test_openai.py
import openai
import os
from dotenv import load_dotenv
load_dotenv()
openai.api_key = os.getenv('OPENAI_API_KEY')
response = openai.Completion.create(
engine="text-davinci-003",
prompt="Hello, world!",
max_tokens=5
)
print(response.choices[0].text.strip())
Running the Script
Activate your virtual environment and execute:
python test_openai.py
If everything is set up correctly, you should see a response from the OpenAI API.
Troubleshooting Common Issues
- Module Not Found Error: Ensure your virtual environment is activated and all packages are installed.
- API Key Error: Double-check that your
.env
file contains the correct API key and that it's being loaded properly. - Permission Issues: On Unix systems, you may need to modify the execution permissions of scripts.
Conclusion
Setting up your development environment is a foundational step that can greatly impact your efficiency and the success of your project. You've now configured Python, set up VS Code with useful extensions, created a virtual environment, and installed the necessary packages. You're ready to start building your chatbot!
In the next article, we'll make our first API call to OpenAI and start interacting with the chatbot capabilities. Stay tuned as we continue this exciting journey.
For more tutorials and insights on boosting your developer productivity, be sure to check out slaptijack.com.