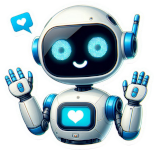
Now that we have our development environment set up, it's time to dive into the exciting part—making our first API call to OpenAI. In this article, we'll walk through obtaining your OpenAI API key, understanding how to authenticate requests, and writing a simple Python script to interact with the API. By the end, you'll have a basic program that can generate text based on a prompt you provide.
Obtaining Your OpenAI API Key
Before you can interact with the OpenAI API, you'll need to sign up for an API key.
Step 1: Sign Up for an Account
- Visit the OpenAI Website: Go to the OpenAI registration page.
- Create an Account: You can sign up using your email address or continue with Google or Microsoft accounts.
- Verify Your Email: OpenAI will send a verification email. Click the link to verify your account.
Step 2: Obtain Your API Key
- Navigate to the API Keys Section: Once logged in, click on your profile icon and select "View API Keys".
- Create a New API Key: Click the "Create new secret key" button.
- Copy the Key: Important—this is the only time you'll be able to view and copy the key. Store it securely.
Step 3: Store the API Key Securely
Add your API key to the .env
file in your project directory:
OPENAI_API_KEY='your-secret-api-key-here'
Remember: Never share your API key publicly or commit it to version control.
Understanding API Authentication and Security Practices
Authentication is handled via the API key in the request header. OpenAI uses HTTPS for all requests, ensuring data is encrypted in transit.
Best Practices
- Use Environment Variables: Store sensitive information like API keys in environment variables.
- Limit Access: Treat your API key like a password. Do not share it.
- Monitor Usage: Keep an eye on your API usage to detect any unauthorized activity.
Writing a Simple Python Script
Let's write a Python script that sends a prompt to the OpenAI API and prints the response.
Step 1: Import Necessary Libraries
import os
import openai
from dotenv import load_dotenv
Step 2: Load Environment Variables
load_dotenv()
openai.api_key = os.getenv('OPENAI_API_KEY')
Step 3: Create the API Call Function
def generate_response(prompt):
response = openai.Completion.create(
engine='text-davinci-003',
prompt=prompt,
max_tokens=50,
n=1,
stop=None,
temperature=0.7,
)
return response.choices[0].text.strip()
Step 4: Test the Function
if __name__ == '__main__':
user_prompt = input("Enter your prompt: ")
reply = generate_response(user_prompt)
print(f"OpenAI says: {reply}")
Full Script: chatbot_simple.py
import os
import openai
from dotenv import load_dotenv
load_dotenv()
openai.api_key = os.getenv('OPENAI_API_KEY')
def generate_response(prompt):
response = openai.Completion.create(
engine='text-davinci-003',
prompt=prompt,
max_tokens=50,
n=1,
stop=None,
temperature=0.7,
)
return response.choices[0].text.strip()
if __name__ == '__main__':
user_prompt = input("Enter your prompt: ")
reply = generate_response(user_prompt)
print(f"OpenAI says: {reply}")
Step 5: Run the Script
Activate your virtual environment and run:
python chatbot_simple.py
Sample Interaction:
Enter your prompt: What is the capital of France?
OpenAI says: The capital of France is Paris.
Understanding the Code
The generate_response
Function
- engine: Specifies the AI model to use.
text-davinci-003
is among the most capable models for natural language tasks. - prompt: The input text that you provide.
- max_tokens: The maximum number of tokens (words or punctuation symbols) in the generated response.
- temperature: Controls the randomness of the output. Lower values make the output more deterministic.
Handling the Response
The API returns a JSON object. We access the generated text via:
response.choices[0].text.strip()
Parsing and Handling API Responses
Understanding the structure of the API response can help you extract more information.
Sample API Response
{
"id": "cmpl-6YzJwLbG5iF7G",
"object": "text_completion",
"created": 1659312021,
"model": "text-davinci-003",
"choices": [
{
"text": "\n\nThe capital of France is Paris.",
"index": 0,
"logprobs": null,
"finish_reason": "length"
}
],
"usage": {
"prompt_tokens": 10,
"completion_tokens": 10,
"total_tokens": 20
}
}
Accessing Additional Information
-
Usage Data: You can access
response.usage
to monitor token usage.print(f"Total tokens used: {response['usage']['total_tokens']}")
-
Finish Reason: Indicates why the completion stopped (e.g., max tokens reached).
print(f"Finish reason: {response.choices[0]['finish_reason']}")
Error Handling
It's essential to handle potential errors gracefully.
Common Errors
- Invalid API Key: Ensure your API key is correct and loaded properly.
- Rate Limits: If you exceed the rate limit, you'll receive an error.
Implementing Error Handling
def generate_response(prompt):
try:
response = openai.Completion.create(
engine='text-davinci-003',
prompt=prompt,
max_tokens=50,
n=1,
stop=None,
temperature=0.7,
)
return response.choices[0].text.strip()
except openai.error.OpenAIError as e:
print(f"An error occurred: {e}")
return None
Best Practices for API Usage
- Optimize Prompts: Be clear and specific to get better responses.
- Monitor Costs: Keep an eye on token usage to manage expenses.
- Cache Responses: If appropriate, cache API responses to reduce redundant calls.
Experimenting with Parameters
Adjusting parameters can significantly affect the output.
- Temperature: Values between 0 (deterministic) and 1 (creative).
- Max Tokens: Controls the length of the response.
- Top P: An alternative to temperature for controlling randomness.
Example: Creative Writing Prompt
response = openai.Completion.create(
engine='text-davinci-003',
prompt='Write a short poem about the sea.',
max_tokens=50,
temperature=0.9,
)
Recommended Tools and Accessories
To enhance your coding and testing experience:
Postman for API Testing
Postman is a powerful tool for testing APIs. It allows you to craft requests and inspect responses without writing code.
Insomnia REST Client
Insomnia is another excellent tool for exploring APIs.
Books on API Design
Consider reading "API Design Patterns" by JJ Geewax to deepen your understanding of API integration.
Conclusion
Congratulations! You've made your first API call to OpenAI and received a generated response. This is a significant step in building your chatbot. Understanding how to interact with APIs is a vital skill in modern software development.
In the next article, we'll build a more interactive command-line interface for our chatbot, allowing for continuous conversation. We'll also delve into handling user input more effectively.
For more tutorials and insights on boosting your developer productivity, be sure to check out slaptijack.com.