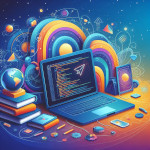
In the previous article, we introduced Lua as a powerful and versatile scripting language. Now, let's dive into the fundamental building blocks that form the foundation of any Lua program: variables, data types, and operators.
1. Variables:
Variables are named storage locations that hold data within your program. You can
think of them as containers that can store different types of information. To
create a variable in Lua, simply assign a value to a name using the equal sign
(=
).
name = "John Doe"
age = 30
Here, name
and age
are variables that store the string "John Doe" and the
number 30, respectively.
2. Data Types:
Lua supports various data types to represent different kinds of information:
- Numbers: Used for numerical values, including integers (e.g., 10, -5) and floating-point numbers (e.g., 3.14, -2.5e-3).
- Strings: Represent sequences of characters enclosed in double quotes (
"
) or single quotes ('
). - Booleans: Represent logical values:
true
orfalse
. - Tables: Powerful data structures that can hold collections of other values, acting like arrays or dictionaries.
- Nil: Represents the absence of a value, similar to
null
in other languages.
3. Operators:
Operators are symbols used to perform various operations on data. Lua provides operators for:
- Arithmetic: Addition (
+
), subtraction (-
), multiplication (*
), division (/
), modulo (%
) - Comparison: Equal to (
==
), not equal to (!=
), greater than (>
), less than (<
), greater than or equal to (>=
), less than or equal to (<=
) - Logical:
and
,or
,not
- Concatenation: Used to join strings (
..
)
Here are some examples of using operators:
result = 10 + 5 -- Addition
age_difference = 30 - 25 -- Subtraction
fullName = "Alice" .. " " .. "Smith" -- String concatenation
isAdult = age >= 18 -- Comparison
Understanding these basic building blocks is crucial for effectively writing Lua programs. In the next article, we will explore control flow statements, allowing your programs to make decisions and execute code conditionally.