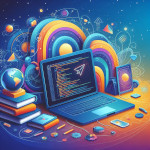
In the previous articles, we explored variables, data types, and operators, the essential building blocks of any Lua program. Now, let's delve into control flow statements, which enable your programs to make decisions and execute code conditionally.
1. Conditional Statements:
Conditional statements allow your program to choose different execution paths based on certain conditions. Lua offers two primary conditional statements:
-
if statement: Evaluates a condition and executes a block of code if the condition is true. Optionally, an
else
block can be used to execute code if the condition is false.age = 20 if age >= 18 then print("You are an adult.") else print("You are not an adult.") end
-
elseif statement: Used within an
if
structure to provide additional conditions to check after the initial condition.grade = 85 if grade >= 90 then print("Excellent!") elseif grade >= 80 then print("Very good!") else print("Keep practicing!") end
2. Loops:
Loops allow you to repeatedly execute a block of code until a certain condition is met. Lua provides two common loop structures:
-
while loop: Executes a block of code as long as a specified condition remains true.
count = 1 while count <= 5 do print(count) count = count + 1 end
-
for loop: Used for iterating over a sequence of values, typically stored in a table.
fruits = {"apple", "banana", "orange"} for _, fruit in ipairs(fruits) do print(fruit) end
Understanding and effectively using control flow statements is essential for writing dynamic and interactive Lua programs. In the next article, we will explore functions, powerful reusable blocks of code that enhance code organization and modularity.