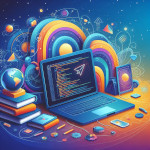
In the previous articles, we covered the fundamentals of variables, data types, operators, and control flow statements, laying the groundwork for building basic Lua programs. Now, let's delve into functions, which are essential building blocks for creating well-structured and reusable code.
1. Defining Functions:
Functions are named blocks of code that perform specific tasks. They encapsulate a set of instructions and can be called upon multiple times throughout your program, promoting code reusability and modularity.
Here's the basic syntax for defining a function in Lua:
function function_name(parameters)
-- Code to be executed when the function is called
return value_to_return
end
- function_name: A unique identifier for your function.
- parameters: (Optional) A comma-separated list of variables that the function can receive as input.
- Code block: The body of the function containing the statements to be executed.
- return statement: (Optional) Used to specify a value to be returned from the function when it finishes execution.
2. Calling Functions:
Once defined, a function can be called by its name followed by parentheses, optionally containing arguments (values passed to the function's parameters).
function greet(name)
print("Hello, " .. name .. "!")
end
greet("Alice") -- Output: Hello, Alice!
3. Benefits of Functions:
Using functions offers several advantages:
- Code Reusability: Functions allow you to write a piece of code once and use it multiple times throughout your program, reducing redundancy and maintaining consistency.
- Modularity: Functions break down complex programs into smaller, manageable units, improving code organization and readability.
- Encapsulation: Functions can encapsulate specific functionalities, hiding internal implementation details and promoting data protection.
4. Advanced Function Features:
Lua supports various advanced features related to functions, including:
- Default arguments: Assigning default values to function parameters in case no arguments are provided during the call.
- Variable number of arguments: Functions can accept a variable number of
arguments using the ellipsis (
...
) notation. - Recursive functions: Functions that can call themselves within their own code block.
Understanding and effectively using functions is crucial for writing well-structured, maintainable, and efficient Lua programs. In the next article, we will explore tables, powerful data structures that can hold various types of information and play a vital role in Lua programming.