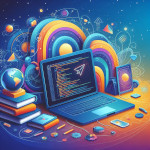
In the previous articles, we covered the core concepts of Lua, including variables, data types, operators, control flow, functions, and tables. Now, let's explore some advanced topics that enhance your ability to write robust and effective Lua programs:
1. Modules and Packages:
- Modules: Reusable blocks of code stored in separate Lua files. They typically export specific functions, variables, or tables that can be used by other parts of your program.
- Packages: Collections of modules organized hierarchically within directories. They provide a structured way to manage and share code across different projects.
2. Loading and Using Modules:
The require
function is used to load and access modules:
-- Load the math module
math = require("math")
-- Use functions from the loaded module
result = math.sqrt(16) -- Output: 4
3. Creating and Sharing Modules:
Modules are typically defined in separate Lua files. You can export specific
elements using the return
statement:
-- my_math.lua
function add(x, y)
return x + y
end
return { add = add } -- Export the 'add' function
This module can be loaded and used in other programs as described earlier.
4. Error Handling:
Lua provides mechanisms for handling errors that occur during program execution. The primary methods include:
error
function: Used to explicitly raise an error with a custom message.pcall
function: Allows you to call a function in "protected mode," catching any errors that occur within the call and returning an error code and message.
function divide(x, y)
if y == 0 then
error("Division by zero!")
end
return x / y
end
success, result = pcall(divide, 10, 0)
if not success then
print(error) -- Handle the error message
else
print(result)
end
5. Conclusion:
Understanding modules, packages, and error handling empowers you to write well-organized, reusable, and robust Lua programs. By effectively utilizing these concepts, you can create complex applications that handle errors gracefully and maintain a clean code structure.
This series has provided a foundational understanding of Lua programming. As you continue your journey, explore additional resources, practice writing your own programs, and delve deeper into advanced topics like metaprogramming and object-oriented programming to further enhance your Lua skills.