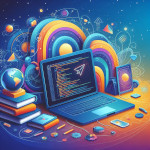
In the previous articles, we explored variables, data types, operators, control flow statements, and functions, equipping you with the essential tools to build basic Lua programs. Now, let's delve into tables, the cornerstone data structure in Lua, offering immense flexibility and functionality.
1. What are Tables?
Tables are associative arrays that can store collections of various data types, acting like both arrays and dictionaries in other languages. They allow you to organize data using keys and values, where keys can be strings, numbers, or even other tables, providing immense flexibility for data representation.
2. Creating Tables:
There are two primary ways to create tables in Lua:
-
Using curly braces: This is the most common method, where you enclose comma-separated key-value pairs within curly braces (
{}
).person = { name = "John Doe", age = 30, city = "New York" }
-
Using the
table
constructor: This method provides more advanced options for table creation, allowing you to specify initial values and custom behavior.shoppingCart = table.new() shoppingCart["apples"] = 3 shoppingCart["bread"] = 1
3. Accessing and Modifying Elements:
You can access individual elements in a table using their keys enclosed in square
brackets ([]
).
name = person["name"] -- Accessing value using string key
age = person[2] -- Accessing value using numeric key (not recommended)
Modifying elements is equally straightforward. Simply assign a new value to the desired key.
person["city"] = "Los Angeles" -- Updating an existing element
shoppingCart["milk"] = 1 -- Adding a new element
4. Iterating over Tables:
Lua provides two common ways to iterate over the elements in a table:
-
ipairs
loop: This loop iterates over the numeric indices of a table, typically used for arrays.for i, fruit in ipairs(fruits) do print(i, fruit) end
-
pairs
loop: This loop iterates over all key-value pairs in a table, regardless of their data types.for key, value in pairs(person) do print(key, value) end
5. Advanced Table Features:
Tables offer various advanced features, including:
- Nested tables: Tables can hold other tables as elements, allowing for hierarchical data structures.
- Table methods: Built-in functions that operate on tables, such as
table.insert
for adding elements andtable.remove
for removing elements. - Metatables: Special tables that control the behavior of other tables, enabling customization and advanced functionalities.
Tables are fundamental to Lua programming and mastering their usage is essential for building efficient and complex data structures. In the next article, we will explore additional topics like modules, packages, and error handling, further expanding your Lua programming skills.